class new in Git master
#include <Magnum/Ui/LineLayer.h>
LineLayer Line layer.
Draws smooth wide lines with configurable caps and joins and per-point colors. Based on the same internal implementation as Shaders::
Setting up a line layer instance
First you need to instantiate LineLayer::3
in the following snippet:
Ui::LineLayerGL::Shared lineLayerShared{ Ui::LineLayer::Shared::Configuration{3} };
The shared instance, in this case a concrete LineLayerGL::
Ui::LineLayer& lineLayer = ui.setLayerInstance( Containers::pointer<Ui::LineLayerGL>(ui.createLayer(), lineLayerShared));
Afterwards, in order to be able to draw the layer, a style has to be set with LineLayer::0
being the default, style 1
being a centered blue wide line and style 2
an azure glow aligned to the bottom left corner of the node:
lineLayerShared.setStyle(Ui::LineLayerCommonStyleUniform{}, { Ui::LineLayerStyleUniform{}, /* Style 0, default */ Ui::LineLayerStyleUniform{} /* Style 1 */ .setColor(0x2f83cc_rgbf) .setWidth(2.0f), Ui::LineLayerStyleUniform{} /* Style 2 */ .setColor(0xa5c9ea_rgbf) .setWidth(3.0f) .setSmoothness(8.0f) }, { Ui::LineAlignment{}, Ui::LineAlignment::MiddleCenter, Ui::LineAlignment::BottomLeft, }, {});
With this, assuming AbstractUserInterface::
Unlike with BaseLayer or TextLayer, the default UserInterface implementation doesn't implicitly provide a LineLayer instance.
Creating lines
A line strip is created by calling createStrip() with desired style index, a list of points that are meant to be connected together and a NodeHandle the data should be attached to. In this case it picks the style 1
from above, which makes a wide blue line:
Ui::NodeHandle node = ui.createNode(…); lineLayer.createStrip(1, { {8.0f, -24.0f}, {8.0f, 24.0f}, {56.0f, 24.0f} }, {}, node);
The createLoop() function then creates a loop, connecting also the first and last point back together. If you give it just a single point, it will render a literal point. The visual output of these three calls is shown below.
lineLayer.createLoop(1, { {-56.0f, -24.0f}, {-8.0f, -24.0f}, {-8.0f, 24.0f}, {-56.0f, 24.0f} }, {}, node); lineLayer.createLoop(1, {{56.0f, -24.0f}}, {}, node);
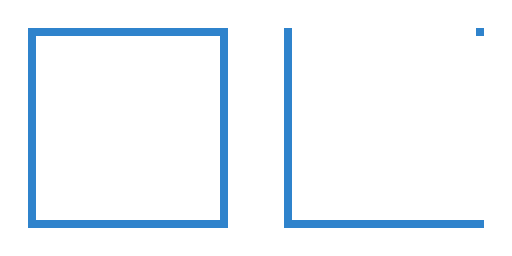
Both createStrip() and createLoop() are convenience alternatives to create(). This function takes an indexed list of points, where each pair of indices describes a line segment. Points that are referenced exactly twice form a line join, if they're referenced twice from a single pair, they form a literal point. The index buffer thus allows you to put multiple disjoint line strips and loops together. An indexed equivalent to the three calls to createStrip() and createLoop() from above would look like this:
lineLayer.create(1, { 0, 1, 1, 2, 3, 4, 4, 5, 5, 6, 6, 3, 7, 7 }, { {8.0f, -24.0f}, {8.0f, 24.0f}, {56.0f, 24.0f}, {-56.0f, -24.0f}, {-8.0f, -24.0f}, {-8.0f, 24.0f}, {-56.0f, 24.0f}, {56.0f, -24.0f} }, {}, node);
As with all other data, they're implicitly tied to lifetime of the node they're attached to. You can remember the Ui::
Style options
Edge smoothness
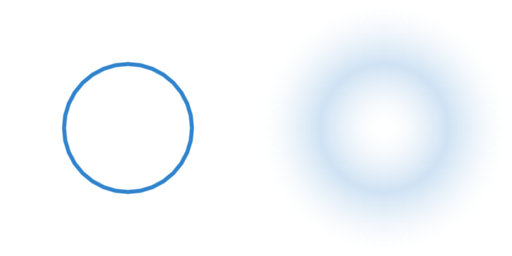
One of the most desirable aspects of line rendering is antialiasing. Like BaseLayer, LineLayer performs it in the shader without needing to rely on multisampling. With LineLayerCommonStyleUniform::
lineLayerShared.setStyle( Ui::LineLayerCommonStyleUniform{} .setSmoothness(1.0f), { Ui::LineLayerStyleUniform{} /* 0 */ .setColor(0x2f83cc_rgbf), Ui::LineLayerStyleUniform{} /* 1 */ .setColor(0xa5c9ea_rgbf) .setSmoothness(6.0f) }, {…}, {}); … Ui::NodeHandle circle = …; lineLayer.createLoop(0, {…}, {}, circle); Ui::NodeHandle glow = …; lineLayer.createLoop(1, {…}, {}, glow);
Line cap and join
The layer allows choosing between various cap and join styles shown below. The default is LineCapStyle::
LineJoinStyle::
If you need to combine lines with different cap and join styles, you can create multiple line layers, each with a differently-configured LineLayer::
Ui::LineLayerGL::Shared lineLayerSharedRound{ Ui::LineLayer::Shared::Configuration{…} .setCapStyle(Ui::LineCapStyle::Round) }; Ui::LineLayerGL::Shared lineLayerSharedSquare{ Ui::LineLayer::Shared::Configuration{…} .setCapStyle(Ui::LineCapStyle::Square) }; Ui::LineLayer& lineLayerRound = ui.setLayerInstance( Containers::pointer<Ui::LineLayerGL>(ui.createLayer(), lineLayerSharedRound)); Ui::LineLayer& lineLayerSquare = ui.setLayerInstance( Containers::pointer<Ui::LineLayerGL>(ui.createLayer(), lineLayerSharedSquare));
Style, per-data and per-point color
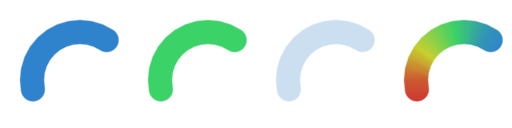
In addition to LineLayerStyleUniform::
lineLayerShared.setStyle(…, { Ui::LineLayerStyleUniform{}, /* 0 */ Ui::LineLayerStyleUniform{} /* 1 */ .setColor(0x2f83cc_rgbf) }, {…}, {}); … Ui::NodeHandle blue = …; lineLayer.createStrip(1, {…}, {}, blue); Ui::NodeHandle colored = …; Ui::DataHandle coloredData = lineLayer.createStrip(0, {…}, {}, colored); lineLayer.setColor(coloredData, 0x3bd267_rgbf); Ui::NodeHandle fadedBlue = …; lineLayer.createStrip(1, {…}, {}, fadedBlue); ui.setNodeOpacity(fadedBlue, 0.25f);
Finally, as visualized with the fourth arc above, it's possible to color each individual point by passing a list of colors to create(), createStrip() or createLoop(), one for each point. The per-point colors then get multiplied with the other colors set for the style and the data.
Ui::NodeHandle gradient = …; lineLayer.createStrip(0, { … }, { 0x2f83cc_rgbf, 0x3bd267_rgbf, 0xc7cf2f_rgbf, … }, gradient);
Alignment and padding inside the node
LineAlignment::{0.0f, 0.0f}
to a vertical and horizontal center of given node, thus as long as the points are aligned around that origin, the whole line art will be centered. At the moment, it's not possible to align based on the actual bounding rectangle of the point data.
LineAlignment::{0.0f, 0.0f}
won't partially leak out of node edges. Similarly as with padding in BaseLayer, it's one value for each of four node edges, but often a single value for all is enough:
lineLayerShared.setStyle(…, { Ui::LineLayerStyleUniform{} .setWidth(5.0f), … }, { Ui::LineAlignment::BottomLeft, … }, { /* Padding is half of line width to make a point at origin touch the bottom left node corner, but not leak out of it */ Vector4{2.5f}, … });
Finally, both alignment and padding can be overriden on a per-data basis with setAlignment() and setPadding(), which may be useful for example when aligning line art next to variable-width text. Note that, however, the draw order isn't guaranteed in case of multiple data attached to the same node, so it only gives a reliable output as long as the shapes don't overlap.
Line width and outline
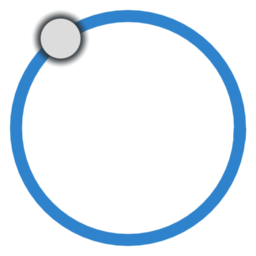
As shown above, line width is controllable with LineLayerStyleUniform::
lineLayerShared.setStyle(…, { Ui::LineLayerStyleUniform{} /* 0 */ .setColor(0x2f83cc_rgbf) .setWidth(3.0f), Ui::LineLayerStyleUniform{} /* 1 */ .setColor(0x292e32_rgbf) .setWidth(11.0f) .setSmoothness(1.5f), Ui::LineLayerStyleUniform{} /* 2 */ .setColor(0xdcdcdc_rgbf) .setWidth(10.0f) }, {…}, {}); Ui::NodeHandle circle = ui.createNode(…); Ui::NodeHandle pointOuter = ui.createNode(circle, …); Ui::NodeHandle point = ui.createNode(pointOuter, …); lineLayer.createLoop(0, {…}, {}, circle); Vector2 position[]{…}; lineLayer.createStrip(1, position, {}, pointOuter); lineLayer.createStrip(2, position, {}, point);
Style transition based on input events
Like with BaseLayer, it's possible to configure LineLayer to perform automatic style transitions based on input events, such as highlighting on hover or press. See the BaseLayer documentation for style transition for a detailed example, the interfaces are the same between the two.
Updating line data
Any created line can be subsequently updated with setLineStrip(), setLineLoop() and setLine(). Those functions take the same arguments and behave the same as as createStrip(), createLoop() and create(), and are a more efficient operation than data removal and recreation if the point and index count doesn't change, which is a common case for example when updating plotted values.
Changing the point / index count is supported however and internally there's also no distinction between a strip, loop or an indexed line, so a strip can be safely changed to a loop etc.
Base classes
- class AbstractVisualLayer new in Git master
- Base for visual data layers.
Derived classes
- class LineLayerGL new in Git master
- OpenGL implementation of the line layer.
Public types
- class Shared
- Shared state for the line layer.
Public functions
- auto shared() -> Shared&
- Shared state used by this layer.
- auto shared() const -> const Shared&
-
auto create(UnsignedInt style,
const Containers::
StridedArrayView1D<const UnsignedInt>& indices, const Containers:: StridedArrayView1D<const Vector2>& points, const Containers:: StridedArrayView1D<const Color4>& colors, NodeHandle node = NodeHandle:: Null) -> DataHandle - Create a line from an indexed list of points.
-
auto create(UnsignedInt style,
std::
initializer_list<UnsignedInt> indices, std:: initializer_list<Vector2> points, std:: initializer_list<Color4> colors, NodeHandle node = NodeHandle:: Null) -> DataHandle -
template<class StyleIndex>auto create(StyleIndex style, const Containers::
StridedArrayView1D<const UnsignedInt>& indices, const Containers:: StridedArrayView1D<const Vector2>& points, const Containers:: StridedArrayView1D<const Color4>& colors, NodeHandle node = NodeHandle:: Null) -> DataHandle - Create a line from an indexed list of points with a style index in a concrete enum type.
-
template<class StyleIndex>auto create(StyleIndex style, std::
initializer_list<UnsignedInt> indices, std:: initializer_list<Vector2> points, std:: initializer_list<Color4> colors, NodeHandle node = NodeHandle:: Null) -> DataHandle -
auto createStrip(UnsignedInt style,
const Containers::
StridedArrayView1D<const Vector2>& points, const Containers:: StridedArrayView1D<const Color4>& colors, NodeHandle node = NodeHandle:: Null) -> DataHandle - Create a line strip.
-
auto createStrip(UnsignedInt style,
std::
initializer_list<Vector2> points, std:: initializer_list<Color4> colors, NodeHandle node = NodeHandle:: Null) -> DataHandle -
template<class StyleIndex>auto createStrip(StyleIndex style, const Containers::
StridedArrayView1D<const Vector2>& points, const Containers:: StridedArrayView1D<const Color4>& colors, NodeHandle node = NodeHandle:: Null) -> DataHandle - Create a line strip with a style index in a concrete enum type.
-
template<class StyleIndex>auto createStrip(StyleIndex style, std::
initializer_list<Vector2> points, std:: initializer_list<Color4> colors, NodeHandle node = NodeHandle:: Null) -> DataHandle -
auto createLoop(UnsignedInt style,
const Containers::
StridedArrayView1D<const Vector2>& points, const Containers:: StridedArrayView1D<const Color4>& colors, NodeHandle node = NodeHandle:: Null) -> DataHandle - Create a line loop.
-
auto createLoop(UnsignedInt style,
std::
initializer_list<Vector2> points, std:: initializer_list<Color4> colors, NodeHandle node = NodeHandle:: Null) -> DataHandle -
template<class StyleIndex>auto createLoop(StyleIndex style, const Containers::
StridedArrayView1D<const Vector2>& points, const Containers:: StridedArrayView1D<const Color4>& colors, NodeHandle node = NodeHandle:: Null) -> DataHandle - Create a line loop with a style index in a concrete enum type.
-
template<class StyleIndex>auto createLoop(StyleIndex style, std::
initializer_list<Vector2> points, std:: initializer_list<Color4> colors, NodeHandle node = NodeHandle:: Null) -> DataHandle - void remove(DataHandle handle)
- Remove a line.
- void remove(LayerDataHandle handle)
- Remove a line assuming it belongs to this layer.
- auto indexCount(DataHandle handle) const -> UnsignedInt
- Line index count.
- auto indexCount(LayerDataHandle handle) const -> UnsignedInt
- Line index count assuming it belongs to this layer.
- auto pointCount(DataHandle handle) const -> UnsignedInt
- Line point count.
- auto pointCount(LayerDataHandle handle) const -> UnsignedInt
- Line point count assuming it belongs to this layer.
-
void setLine(DataHandle handle,
const Containers::
StridedArrayView1D<const UnsignedInt>& indices, const Containers:: StridedArrayView1D<const Vector2>& points, const Containers:: StridedArrayView1D<const Color4>& colors) - Set line data.
-
void setLine(DataHandle handle,
std::
initializer_list<UnsignedInt> indices, std:: initializer_list<Vector2> points, std:: initializer_list<Color4> colors) -
void setLine(LayerDataHandle handle,
const Containers::
StridedArrayView1D<const UnsignedInt>& indices, const Containers:: StridedArrayView1D<const Vector2>& points, const Containers:: StridedArrayView1D<const Color4>& colors) - Set line data assuming it belongs to this layer.
-
void setLine(LayerDataHandle handle,
std::
initializer_list<UnsignedInt> indices, std:: initializer_list<Vector2> points, std:: initializer_list<Color4> colors) -
void setLineStrip(DataHandle handle,
const Containers::
StridedArrayView1D<const Vector2>& points, const Containers:: StridedArrayView1D<const Color4>& colors) - Set line strip data.
-
void setLineStrip(DataHandle handle,
std::
initializer_list<Vector2> points, std:: initializer_list<Color4> colors) -
void setLineStrip(LayerDataHandle handle,
const Containers::
StridedArrayView1D<const Vector2>& points, const Containers:: StridedArrayView1D<const Color4>& colors) - Set line strip data assuming it belongs to this layer.
-
void setLineStrip(LayerDataHandle handle,
std::
initializer_list<Vector2> points, std:: initializer_list<Color4> colors) -
void setLineLoop(DataHandle handle,
const Containers::
StridedArrayView1D<const Vector2>& points, const Containers:: StridedArrayView1D<const Color4>& colors) - Set line loop data.
-
void setLineLoop(DataHandle handle,
std::
initializer_list<Vector2> points, std:: initializer_list<Color4> colors) -
void setLineLoop(LayerDataHandle handle,
const Containers::
StridedArrayView1D<const Vector2>& points, const Containers:: StridedArrayView1D<const Color4>& colors) - Set line loop data assuming it belongs to this layer.
-
void setLineLoop(LayerDataHandle handle,
std::
initializer_list<Vector2> points, std:: initializer_list<Color4> colors) - auto color(DataHandle handle) const -> Color4
- Custom line color.
- auto color(LayerDataHandle handle) const -> Color4
- Custom line color assuming it belongs to this layer.
- void setColor(DataHandle handle, const Color4& color)
- Set custom line color.
- void setColor(LayerDataHandle handle, const Color4& color)
- Set custom line color assuming it belongs to this layer.
-
auto alignment(DataHandle handle) const -> Containers::
Optional<LineAlignment> - Custom line alignment.
-
auto alignment(LayerDataHandle handle) const -> Containers::
Optional<LineAlignment> - Custom line alignment assuming it belongs to this layer.
-
void setAlignment(DataHandle handle,
Containers::
Optional<LineAlignment> alignment) - Set custom line alignment.
-
void setAlignment(LayerDataHandle handle,
Containers::
Optional<LineAlignment> alignment) - Set custom line alignment assuming it belongs to this layer.
- auto padding(DataHandle handle) const -> Vector4
- Custom line padding.
- auto padding(LayerDataHandle handle) const -> Vector4
- Custom line padding assuming it belongs to this layer.
- void setPadding(DataHandle handle, const Vector4& padding)
- Set custom line padding.
- void setPadding(LayerDataHandle handle, const Vector4& padding)
- Set custom line padding assuming it belongs to this layer.
- void setPadding(DataHandle handle, Float padding)
- Set custom line padding with all edges having the same value.
- void setPadding(LayerDataHandle handle, Float padding)
- Set custom line padding with all edges having the same value assuming it belongs to this layer.
Function documentation
Shared& Magnum:: Ui:: LineLayer:: shared()
Shared state used by this layer.
Reference to the instance passed to LineLayerGL::
DataHandle Magnum:: Ui:: LineLayer:: create(UnsignedInt style,
const Containers:: StridedArrayView1D<const UnsignedInt>& indices,
const Containers:: StridedArrayView1D<const Vector2>& points,
const Containers:: StridedArrayView1D<const Color4>& colors,
NodeHandle node = NodeHandle:: Null)
Create a line from an indexed list of points.
Parameters | |
---|---|
style | Style index |
indices | Indices pointing into the points view |
points | Line points indexed by indices |
colors | Optional per-point colors |
node | Node to attach to |
Returns | New data handle |
Expects that style
is less than Shared::style
.
The indices
are expected to have an even size and their values are all expected to be less than size of points
. Every successive pair of values describes one line segment. If the same index is used exactly twice in two different segments, it's drawn as a line join, otherwise it's drawn as a line cap. A pair of the same values draws a point. For example, assuming the points
array has at least 7 items, the following sequence of indices draws a closed line loop, a single line segment and a point:
0, 1, 1, 2, 2, 3, 3, 0, // loop with four segments 4, 5, // standalone line segment with two caps 6, 6 // a single point
Note that the only purpose of the index buffer is to describe connections between line points and for rendering the lines get converted to a different representation. It's not an error if the index buffer doesn't reference all points
, it's also not an error if the same point is present more than once.
The colors
array is expected to be either empty or have the same size as points
. If non-empty, each point is drawn with a corresponding color that's further multiplied by a color coming from the style and potentially from setColor(). If empty, it's as if an array of 0xffffffff_srgbaf
was supplied.
DataHandle Magnum:: Ui:: LineLayer:: create(UnsignedInt style,
std:: initializer_list<UnsignedInt> indices,
std:: initializer_list<Vector2> points,
std:: initializer_list<Color4> colors,
NodeHandle node = NodeHandle:: Null)
This is an overloaded member function, provided for convenience. It differs from the above function only in what argument(s) it accepts.
template<class StyleIndex>
DataHandle Magnum:: Ui:: LineLayer:: create(StyleIndex style,
const Containers:: StridedArrayView1D<const UnsignedInt>& indices,
const Containers:: StridedArrayView1D<const Vector2>& points,
const Containers:: StridedArrayView1D<const Color4>& colors,
NodeHandle node = NodeHandle:: Null)
Create a line from an indexed list of points with a style index in a concrete enum type.
Casts style
to UnsignedInt and delegates to create(UnsignedInt, const Containers::
template<class StyleIndex>
DataHandle Magnum:: Ui:: LineLayer:: create(StyleIndex style,
std:: initializer_list<UnsignedInt> indices,
std:: initializer_list<Vector2> points,
std:: initializer_list<Color4> colors,
NodeHandle node = NodeHandle:: Null)
This is an overloaded member function, provided for convenience. It differs from the above function only in what argument(s) it accepts.
DataHandle Magnum:: Ui:: LineLayer:: createStrip(UnsignedInt style,
const Containers:: StridedArrayView1D<const Vector2>& points,
const Containers:: StridedArrayView1D<const Color4>& colors,
NodeHandle node = NodeHandle:: Null)
Create a line strip.
Parameters | |
---|---|
style | Style index |
points | Line strip points |
colors | Optional per-point colors |
node | Node to attach to |
Returns | New data handle |
Creates a single connected line strip. The points
are expected to be either empty or at least two. Convenience equivalent to calling create(UnsignedInt, const Containers::indices
being a {0, 1, 1, 2, 2, 3, ..., points.size() - 2, points.size() - 1}
range. See its documentation for more information about other arguments.
DataHandle Magnum:: Ui:: LineLayer:: createStrip(UnsignedInt style,
std:: initializer_list<Vector2> points,
std:: initializer_list<Color4> colors,
NodeHandle node = NodeHandle:: Null)
This is an overloaded member function, provided for convenience. It differs from the above function only in what argument(s) it accepts.
template<class StyleIndex>
DataHandle Magnum:: Ui:: LineLayer:: createStrip(StyleIndex style,
const Containers:: StridedArrayView1D<const Vector2>& points,
const Containers:: StridedArrayView1D<const Color4>& colors,
NodeHandle node = NodeHandle:: Null)
Create a line strip with a style index in a concrete enum type.
Casts style
to UnsignedInt and delegates to createStrip(UnsignedInt, const Containers::
template<class StyleIndex>
DataHandle Magnum:: Ui:: LineLayer:: createStrip(StyleIndex style,
std:: initializer_list<Vector2> points,
std:: initializer_list<Color4> colors,
NodeHandle node = NodeHandle:: Null)
This is an overloaded member function, provided for convenience. It differs from the above function only in what argument(s) it accepts.
DataHandle Magnum:: Ui:: LineLayer:: createLoop(UnsignedInt style,
const Containers:: StridedArrayView1D<const Vector2>& points,
const Containers:: StridedArrayView1D<const Color4>& colors,
NodeHandle node = NodeHandle:: Null)
Create a line loop.
Parameters | |
---|---|
style | Style index |
points | Line loop points |
colors | Optional per-point colors |
node | Node to attach to |
Returns | New data handle |
Creates a single line loop with the last point connected to the first. The points
are expected to be either empty, a single point (which will create a literal point) or at least three. Convenience equivalent to calling create(UnsignedInt, const Containers::indices
being a {0, 1, 1, 2, 2, 3, ..., points.size() - 1, 0,
range. See its documentation for more information about other arguments.
DataHandle Magnum:: Ui:: LineLayer:: createLoop(UnsignedInt style,
std:: initializer_list<Vector2> points,
std:: initializer_list<Color4> colors,
NodeHandle node = NodeHandle:: Null)
This is an overloaded member function, provided for convenience. It differs from the above function only in what argument(s) it accepts.
template<class StyleIndex>
DataHandle Magnum:: Ui:: LineLayer:: createLoop(StyleIndex style,
const Containers:: StridedArrayView1D<const Vector2>& points,
const Containers:: StridedArrayView1D<const Color4>& colors,
NodeHandle node = NodeHandle:: Null)
Create a line loop with a style index in a concrete enum type.
Casts style
to UnsignedInt and delegates to createLoop(UnsignedInt, const Containers::
template<class StyleIndex>
DataHandle Magnum:: Ui:: LineLayer:: createLoop(StyleIndex style,
std:: initializer_list<Vector2> points,
std:: initializer_list<Color4> colors,
NodeHandle node = NodeHandle:: Null)
This is an overloaded member function, provided for convenience. It differs from the above function only in what argument(s) it accepts.
void Magnum:: Ui:: LineLayer:: remove(DataHandle handle)
Remove a line.
Delegates to AbstractLayer::
void Magnum:: Ui:: LineLayer:: remove(LayerDataHandle handle)
Remove a line assuming it belongs to this layer.
Delegates to AbstractLayer::
UnsignedInt Magnum:: Ui:: LineLayer:: indexCount(DataHandle handle) const
Line index count.
Count of indices passed to create() or setLine(). In case of createStrip() / setLineStrip() the count is 2*pointCount - 2
, in case of createLoop() / setLineLoop() the count is 2*pointCount
. Expects that handle
is valid.
UnsignedInt Magnum:: Ui:: LineLayer:: indexCount(LayerDataHandle handle) const
Line index count assuming it belongs to this layer.
Like indexCount(DataHandle) const but without checking that handle
indeed belongs to this layer. See its documentation for more information.
UnsignedInt Magnum:: Ui:: LineLayer:: pointCount(DataHandle handle) const
Line point count.
Count of points passed to create(), createStrip(), createLoop(), setLine(), setLineStrip() or setLineLoop(). Expects that handle
is valid.
UnsignedInt Magnum:: Ui:: LineLayer:: pointCount(LayerDataHandle handle) const
Line point count assuming it belongs to this layer.
Like pointCount(DataHandle) const but without checking that handle
indeed belongs to this layer. See its documentation for more information.
void Magnum:: Ui:: LineLayer:: setLine(DataHandle handle,
const Containers:: StridedArrayView1D<const UnsignedInt>& indices,
const Containers:: StridedArrayView1D<const Vector2>& points,
const Containers:: StridedArrayView1D<const Color4>& colors)
Set line data.
Expects that handle
is valid. The indices
, points
and colors
are interpreted the same way with the same restrictions as in create(UnsignedInt, const Containers::
Calling this function causes LayerState::
void Magnum:: Ui:: LineLayer:: setLine(DataHandle handle,
std:: initializer_list<UnsignedInt> indices,
std:: initializer_list<Vector2> points,
std:: initializer_list<Color4> colors)
This is an overloaded member function, provided for convenience. It differs from the above function only in what argument(s) it accepts.
void Magnum:: Ui:: LineLayer:: setLine(LayerDataHandle handle,
const Containers:: StridedArrayView1D<const UnsignedInt>& indices,
const Containers:: StridedArrayView1D<const Vector2>& points,
const Containers:: StridedArrayView1D<const Color4>& colors)
Set line data assuming it belongs to this layer.
Like setLine(DataHandle, const Containers::handle
indeed belongs to this layer. See its documentation for more information.
void Magnum:: Ui:: LineLayer:: setLine(LayerDataHandle handle,
std:: initializer_list<UnsignedInt> indices,
std:: initializer_list<Vector2> points,
std:: initializer_list<Color4> colors)
This is an overloaded member function, provided for convenience. It differs from the above function only in what argument(s) it accepts.
void Magnum:: Ui:: LineLayer:: setLineStrip(DataHandle handle,
const Containers:: StridedArrayView1D<const Vector2>& points,
const Containers:: StridedArrayView1D<const Color4>& colors)
Set line strip data.
Expects that handle
is valid. The points
and colors
are interpreted the same way with the same restrictions as in createStrip(UnsignedInt, const Containers::
Calling this function causes LayerState::
void Magnum:: Ui:: LineLayer:: setLineStrip(DataHandle handle,
std:: initializer_list<Vector2> points,
std:: initializer_list<Color4> colors)
This is an overloaded member function, provided for convenience. It differs from the above function only in what argument(s) it accepts.
void Magnum:: Ui:: LineLayer:: setLineStrip(LayerDataHandle handle,
const Containers:: StridedArrayView1D<const Vector2>& points,
const Containers:: StridedArrayView1D<const Color4>& colors)
Set line strip data assuming it belongs to this layer.
Like setLineStrip(DataHandle, const Containers::handle
indeed belongs to this layer. See its documentation for more information.
void Magnum:: Ui:: LineLayer:: setLineStrip(LayerDataHandle handle,
std:: initializer_list<Vector2> points,
std:: initializer_list<Color4> colors)
This is an overloaded member function, provided for convenience. It differs from the above function only in what argument(s) it accepts.
void Magnum:: Ui:: LineLayer:: setLineLoop(DataHandle handle,
const Containers:: StridedArrayView1D<const Vector2>& points,
const Containers:: StridedArrayView1D<const Color4>& colors)
Set line loop data.
Expects that handle
is valid. The points
and colors
are interpreted the same way with the same restrictions as in createLoop(UnsignedInt, const Containers::
Calling this function causes LayerState::
void Magnum:: Ui:: LineLayer:: setLineLoop(DataHandle handle,
std:: initializer_list<Vector2> points,
std:: initializer_list<Color4> colors)
This is an overloaded member function, provided for convenience. It differs from the above function only in what argument(s) it accepts.
void Magnum:: Ui:: LineLayer:: setLineLoop(LayerDataHandle handle,
const Containers:: StridedArrayView1D<const Vector2>& points,
const Containers:: StridedArrayView1D<const Color4>& colors)
Set line loop data assuming it belongs to this layer.
Like setLineLoop(DataHandle, const Containers::handle
indeed belongs to this layer. See its documentation for more information.
void Magnum:: Ui:: LineLayer:: setLineLoop(LayerDataHandle handle,
std:: initializer_list<Vector2> points,
std:: initializer_list<Color4> colors)
This is an overloaded member function, provided for convenience. It differs from the above function only in what argument(s) it accepts.
Color4 Magnum:: Ui:: LineLayer:: color(DataHandle handle) const
Custom line color.
Expects that handle
is valid.
Color4 Magnum:: Ui:: LineLayer:: color(LayerDataHandle handle) const
Custom line color assuming it belongs to this layer.
Expects that handle
is valid.
void Magnum:: Ui:: LineLayer:: setColor(DataHandle handle,
const Color4& color)
Set custom line color.
Expects that handle
is valid. LineLayerStyleUniform::color
. By default, the custom color is 0xffffffff_srgbaf
, i.e. not affecting the style or per-point colors in any way.
Calling this function causes LayerState::
void Magnum:: Ui:: LineLayer:: setColor(LayerDataHandle handle,
const Color4& color)
Set custom line color assuming it belongs to this layer.
Like setColor(DataHandle, const Color4&) but without checking that handle
indeed belongs to this layer. See its documentation for more information.
Containers:: Optional<LineAlignment> Magnum:: Ui:: LineLayer:: alignment(DataHandle handle) const
Custom line alignment.
Expects that handle
is valid. If Containers::
Containers:: Optional<LineAlignment> Magnum:: Ui:: LineLayer:: alignment(LayerDataHandle handle) const
Custom line alignment assuming it belongs to this layer.
Expects that handle
is valid. If Containers::
void Magnum:: Ui:: LineLayer:: setAlignment(DataHandle handle,
Containers:: Optional<LineAlignment> alignment)
Set custom line alignment.
Expects that handle
is valid. Setting the alignment to Containers::
Calling this function causes LayerState::
void Magnum:: Ui:: LineLayer:: setAlignment(LayerDataHandle handle,
Containers:: Optional<LineAlignment> alignment)
Set custom line alignment assuming it belongs to this layer.
Like setAlignment(DataHandle, Containers::handle
indeed belongs to this layer. See its documentation for more information.
Vector4 Magnum:: Ui:: LineLayer:: padding(DataHandle handle) const
Custom line padding.
In order left, top. right, bottom. Expects that handle
is valid.
Vector4 Magnum:: Ui:: LineLayer:: padding(LayerDataHandle handle) const
Custom line padding assuming it belongs to this layer.
In order left, top. right, bottom. Expects that handle
is valid.
void Magnum:: Ui:: LineLayer:: setPadding(DataHandle handle,
const Vector4& padding)
Set custom line padding.
Expects that handle
is valid. The padding
is in order left, top, right, bottom and is added to the per-style padding values specified in Shared::
Calling this function causes LayerState::
void Magnum:: Ui:: LineLayer:: setPadding(LayerDataHandle handle,
const Vector4& padding)
Set custom line padding assuming it belongs to this layer.
Like setPadding(DataHandle, const Vector4&) but without checking that handle
indeed belongs to this layer. See its documentation for more information.
void Magnum:: Ui:: LineLayer:: setPadding(DataHandle handle,
Float padding)
Set custom line padding with all edges having the same value.
Expects that handle
is valid. The padding
is added to the per-style padding values specified in Shared::
Calling this function causes LayerState::
void Magnum:: Ui:: LineLayer:: setPadding(LayerDataHandle handle,
Float padding)
Set custom line padding with all edges having the same value assuming it belongs to this layer.
Like setPadding(DataHandle, Float) but without checking that handle
indeed belongs to this layer. See its documentation for more information.