class new in Git master
#include <Magnum/Ui/TextLayer.h>
TextLayer Text layer.
Draws text laid out using the Text library, including editing capabilities.
Setting up a text layer instance
If you create a UserInterfaceGL with a style and don't exclude StyleFeature::
For a custom layer, you first need to instantiate TextLayer::
Text::GlyphCacheArrayGL glyphCache{PixelFormat::R8Unorm, {256, 256, 4}};
The glyph cache is expected to be alive for the whole shared instance lifetime, alternatively you can use the TextLayerGL::3
in the following snippet:
Ui::TextLayerGL::Shared textLayerShared{glyphCache, Ui::TextLayer::Shared::Configuration{3} };
The shared instance, in this case a concrete TextLayerGL::
ui.setTextLayerInstance( Containers::pointer<Ui::TextLayerGL>(ui.createLayer(), textLayerShared));
Otherwise, if you want to set up a custom base layer that's independent of the one exposed through UserInterface::
Ui::TextLayer& textLayer = ui.setLayerInstance( Containers::pointer<Ui::TextLayerGL>(ui.createLayer(), textLayerShared));
Afterwards, in order to be able to draw the layer, at least one font has to be added, and a style referencing the fonts has to be set. Text::
PluginManager::Manager<Text::AbstractFont> fontManager; Containers::Pointer<Text::AbstractFont> font16 = fontManager.loadAndInstantiate("TrueTypeFont"); if(!font16 || !font16->openFile("font.ttf", 16.0f) || !font16->fillGlyphCache(glyphCache, "abcdefghijklmnopqrstuvwxyz" "ABCDEFGHIJKLMNOPQRSTUVWXYZ" "0123456789?!:;,. ")) Fatal{} << "Uh oh"; Containers::Pointer<Text::AbstractFont> font12 = fontManager.loadAndInstantiate("TrueTypeFont"); if(!font12 || !font12->openFile("font.ttf", 12.0f) || !font12->fillGlyphCache(glyphCache, "abcdefghijklmnopqrstuvwxyz" "ABCDEFGHIJKLMNOPQRSTUVWXYZ" "0123456789?!:;,. ")) Fatal{} << "Oh no"; Ui::FontHandle font16Handle = textLayerShared.addFont(*font16, font16->size()); Ui::FontHandle font12Handle = textLayerShared.addFont(*font12, font12->size());
Assuming the UI size matches the framebuffer size, a good default is to use the same size in Text::
Finally, a style referencing the fonts has to be set with TextLayer::0
uses the first, larger font and style defaults, style 1
is the first font again but with a blue color, and style 2
is the smaller font centered:
textLayerShared.setStyle(Ui::TextLayerCommonStyleUniform{}, { Ui::TextLayerStyleUniform{}, /* Style 0, default */ Ui::TextLayerStyleUniform{} /* Style 1 */ .setColor(0x2f83cc_rgbf), Ui::TextLayerStyleUniform{} /* Style 2 */ }, { font16Handle, /* Font for style 0 */ font16Handle, /* Font for style 1 */ font12Handle /* Font for style 2 */ }, { Text::Alignment{}, /* Alignment for style 0 */ Text::Alignment{}, /* Alignment for style 1 */ Text::Alignment::MiddleCenter /* Alignment for style 2 */ }, {}, {}, {}, {}, {}, {});
Similarly as with BaseLayer styles, to have it easier to match data belonging to the same style, you can make a custom struct
and make views on individual elements using Containers::
Ui::TextLayerStyleUniform uniforms[]{ Ui::TextLayerStyleUniform{}, /* Style 0 */ Ui::TextLayerStyleUniform{} /* Style 1 */ .setColor(0x2f83cc_rgbf), Ui::TextLayerStyleUniform{} /* Style 2 */ }; struct Style { Ui::FontHandle fontHandle; Text::Alignment alignment; } styles[]{ {font16Handle, Text::Alignment{}}, /* Font & alignment for style 0 */ {font16Handle, Text::Alignment{}}, /* Font & alignment for style 1 */ {font12Handle, Text::Alignment::MiddleCenter}, /* ... for style 2 */ }; textLayerShared.setStyle(…, uniforms, Containers::stridedArrayView(styles).slice(&Style::fontHandle), Containers::stridedArrayView(styles).slice(&Style::alignment), {}, {}, {}, {}, {}, {});
With this, assuming AbstractUserInterface::
Creating texts
A text is created by calling create() with desired style index, the actual UTF-8 string to render, a TextProperties instance with optional data-specific shaping and layout settings, and a NodeHandle the data should be attached to. In this case it picks the style 1
from above, which makes the text blue:
Ui::NodeHandle node = ui.createNode(…); textLayer.create(1, "hello!", {}, node);
As with all other data, they're implicitly tied to lifetime of the node they're attached to. You can remember the DataHandle returned by create() to modify the data later, attach() to a different node or remove() it.
Style, shaping and layout options
Text color

The color supplied with TextLayerStyleUniform::
textLayerShared.setStyle(…, { Ui::TextLayerStyleUniform{}, /* 0 */ Ui::TextLayerStyleUniform{} /* 1 */ .setColor(0x2f83cc_rgbf) }, {…}, {…}, {}, {}, {}, {}, {}, {}); … Ui::NodeHandle blue = …; textLayer.create(1, "hello!", {}, blue); Ui::NodeHandle colored = …; Ui::DataHandle coloredData = textLayer.create(0, "HEY", {}, colored); textLayer.setColor(coloredData, 0x3bd267_rgbf); Ui::NodeHandle fadedBlue = …; textLayer.create(1, "shh", {}, fadedBlue); ui.setNodeOpacity(fadedBlue, 0.25f);
At the moment, there's no possibility to assign different color to individual glyphs or text ranges.
Alignment and padding inside the node
Text::
textLayerShared.setStyle(…, {…}, {…}, { Text::Alignment::TopLeft }, {}, {}, {}, {}, {}, { Vector4{8.0f} });
If needed, style-provided alignment can be overriden for particular data with TextProperties::
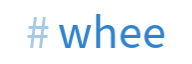
Finally, the padding can be overriden on a per-data basis with setPadding(). This is especially useful when aligning text of a variable width next to other UI elements such as icons, or combining two differently styled pieces of text together. The following snippet shows aligning a text next to a colored hash character using size() to query the actual rendered size, the other element could also be anything from a BaseLayer or a LineLayer which provide the same padding interfaces. Additionally, the space between the two is defined by the style itself and thus doesn't have to be hardcoded in the offset calculation:
textLayerShared.setStyle(…, { Ui::TextLayerStyleUniform{} /* 0 */ .setColor(0xa5c9ea_rgbf), Ui::TextLayerStyleUniform{} /* 1 */ .setColor(0x2f83cc_rgbf) }, {…}, { /* With centered alignment half of the padding value is used for each */ Text::Alignment::MiddleCenter, Text::Alignment::MiddleCenter }, {}, {}, {}, {}, {}, { /* Left, top, right, bottom */ {0.0f, 0.0f, 2.0f, 0.0f}, {2.0f, 0.0f, 0.0f, 0.0f} }); … Ui::NodeHandle node = …; Ui::DataHandle hash = textLayer.create(0, "#", {}, node); Ui::DataHandle text = textLayer.create(1, "whee", {}, node); /* Left, top, right, bottom. Again, centered alignment makes use of half of the padding value for each, keeping the two centered as a group. */ textLayer.setPadding(hash, {0.0f, 0.0f, textLayer.size(text).x(), 0.0f}); textLayer.setPadding(text, {textLayer.size(hash).x(), 0.0f, 0.0f, 0.0f});
Font, shaping language, script and direction
Besides alignment, TextProperties::
Ui::FontHandle greekFontHandle = textLayerShared.addFont(…); … Ui::NodeHandle hello = …; textLayer.create(…, "Γεια!", greekFontHandle, hello);
It's also possible to explicitly specify the script, language and direction of given text, however note that support for these options is highly dependent on the font plugin used. HarfBuzzFont, for example, attempts to detect these properties by default, so not setting them to any fixed value will likely provide better results when working with text coming from unknown international sources. On the other hand, specifying these properties if you're sure about the text origin avoids the autodetection, which can improve performance with highly dynamic text. In contrast, StbTrueTypeFont for example doesn't support any of these properties and setting them will have no effect.
textLayer.create(…, "Γεια!", Ui::TextProperties{} .setFont(greekFontHandle) .setScript(Text::Script::Greek) .setLanguage("el-GR") .setShapeDirection(Text::ShapeDirection::LeftToRight), hello);
At the moment, only Text::
Font feature selection
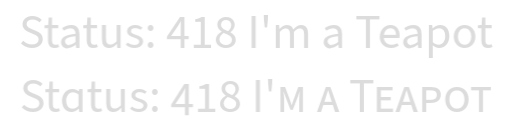
TextProperties::
textLayer.create(…, "Status: 418 I'm a Teapot", Ui::TextProperties{}.setFeatures({ Text::Feature::OldstyleFigures, {Text::Feature::CharacterVariants2, 2, 3}, {Text::Feature::SmallCapitals, 8, ~UnsignedInt{}}, }), …);
Note that the features will likely need additional glyphs to be pre-filled in the cache. The Text::
font->fillGlyphCache(glyphCache, { /* Small capitals */ font->glyphForName("A.s"), font->glyphForName("B.s"), font->glyphForName("C.s"), font->glyphForName("D.s"), … /* Oldstyle figures */ font->glyphForName("one.t"), font->glyphForName("two.t"), font->glyphForName("three.t"), … /* Character variant for lowercase a */ font->glyphForName("a.a") });
Font features can be also specified as part of the style. For example you may want to have all title labels in small caps, and all numeric fields with Text::0
means no features are used for given style:
textLayerShared.setStyle(…, { Ui::TextLayerStyleUniform{} /* title */ .setColor(0xdcdcdc_rgbf), Ui::TextLayerStyleUniform{} /* main title */ .setColor(0xa5c9ea_rgbf), Ui::TextLayerStyleUniform{} /* numeric fields */ .setColor(0xdcdcdc_rgbf), Ui::TextLayerStyleUniform{} /* general text */ .setColor(0xdcdcdc_rgbf) }, {…}, {…}, { Text::Feature::SmallCapitals, /* 0 */ Text::Feature::TabularFigures, /* 1 */ Text::Feature::SlashedZero, /* 2 */ }, { 0, /* title */ 0, /* main title */ 1, /* numeric fields */ 0, /* general text */ }, { 1, /* title uses feature 0 */ 1, /* main title uses feature 0 as well */ 2, /* numeric fields use feature 1 and 2 */ 0 /* general text uses no features */ }, {}, {}, {});
Text crispness and DPI awareness
With Text::
/* Supersample 2x in addition to DPI scaling */ Float scale = 2.0f*(Vector2{ui.framebufferSize()}/ui.size()).max(); font->openFile("font.ttf", 16.0f); … Ui::FontHandle fontHandle = textLayerShared.addFont(*font, font->size()*scale);
Fonts added with TextLayerGL::scale
directly.
Distance field rendering
With Text::
Having a dedicated font for every size becomes impractical if the application needs to use many font sizes, if it needs to scale the text dynamically or if advanced effects such as thinning, thickening or outline are needed. For such cases, it's possible to use Text::{256, 256}
Text::{1024, 1024}
source image for the distance field should use a 64 pt font. The radius should then be chosen so it's at least one or two pixels in the scaled-down result, so in this case at least 4
:
Text::DistanceFieldGlyphCacheArrayGL glyphCache{{1024, 1024, 4}, {256, 256}, 20}; Ui::TextLayerGL::Shared textLayerShared{glyphCache, Ui::TextLayer::Shared::Configuration{3} };
The glyph cache is expected to be alive for the whole shared instance lifetime, or you can again use the TextLayerGL::
Containers::Pointer<Text::AbstractFont> font = fontManager.loadAndInstantiate("TrueTypeFont"); font->openFile("font.ttf", 64.0f); font->fillGlyphCache(glyphCache, "abcdefghijklmnopqrstuvwxyz" "ABCDEFGHIJKLMNOPQRSTUVWXYZ" "0123456789?!:;,. "); Ui::FontHandle font8Handle = textLayerShared.addFont(*font, 8.0f); Ui::FontHandle font16Handle = textLayerShared.addFont(*font, 16.0f); Ui::FontHandle font12Handle = textLayerShared.addFont(*font, 12.0f);
Edge smoothness
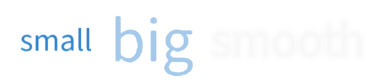
With Text::
textLayerShared.setStyle( Ui::TextLayerCommonStyleUniform{} .setSmoothness(1.0f), {Ui::TextLayerStyleUniform{} /* 0 */ .setColor(0x2f83cc_rgbf), Ui::TextLayerStyleUniform{} /* 1 */ .setColor(0xa5c9ea_rgbf), Ui::TextLayerStyleUniform{} /* 2 */ .setColor(0xdcdcdc_rgbf) .setSmoothness(4.0f)}, {font8Handle, /* Font for style 0 */ font16Handle, /* Font for style 1 */ font12Handle}, /* Font for style 2 */ {…}, {}, {}, {}, {}, {}, {}); … Ui::NodeHandle small = …; Ui::NodeHandle big = …; Ui::NodeHandle smooth = …; textLayer.create(0, "small", {}, small); textLayer.create(1, "big", {}, big); textLayer.create(2, "smooth", {}, smooth);
Note that the smoothness is not depending on the size at which the text is actually drawn, it's always in pixels or UI units.
Edge offset and outline

TextLayerStyleUniform::
textLayerShared.setStyle(…, { Ui::TextLayerStyleUniform{} /* 0 */ .setColor(0xdcdcdc_rgbf) .setOutlineColor(0x2f83cc_rgbf) .setOutlineWidth(1.25f) .setEdgeOffset(0.625f), Ui::TextLayerStyleUniform{} /* 1 */ .setColor(0x2f83cc_rgbf) .setEdgeOffset(0.75f) }, {…}, {…}, {}, {}, {}, {}, {}, {}); … Ui::NodeHandle edgy = …; Ui::NodeHandle bulky = …; textLayer.create(0, "edgy.", {}, edgy); textLayer.create(1, "bulky?!", {}, bulky);
The range how far an outline together with edge offset can go is clamped to the radius used when constructing the Text::20
, together with the font being scaled down from 64.0f
, means the edge can extend at most five pixels for font16Handle
or two and half for font8Handle
. If you don't need that much, you can reduce the radius, which also means the glyphs will occupy less space and the distance field processing takes less time.
Similarly as with the smoothness, the outline width and offset doesn't depend on size of the font actually used in given style. Consistently with BaseLayer outline drawing the outline color isn't affected by setColor().
Rendering single glyphs

With createGlyph() you can render a single glyph specified with its ID. This is useful mainly with icon fonts, where you can for example use Text::
textLayer.createGlyph(…, font->glyphForName("coffee"), {}, node);
Or you can maintain an enum
with name-to-glyph-ID mapping. Similarly to style IDs, createGlyph() accepts both plain integers and enums for a glyph ID:
enum class IconFont: UnsignedInt { … Coffee = 2249, /* queried from the font in an offline step */ }; textLayer.createGlyph(…, IconFont::Coffee, {}, node);
It's however also possible to insert your own image data into the glyph cache and reference them with this function. Assuming a list of images imported for example from a set of PNGs, call Text::
Containers::Array<Trade::ImageData2D> icons = …; /* or Image, ImageView, etc. */ UnsignedInt iconFontId = textLayerShared.glyphCache().addFont(icons.size()); Containers::Array<Vector3i> offsets{NoInit, icons.size()}; Containers::Optional<Range3Di> flushRange = textLayerShared.glyphCache().atlas() .add(stridedArrayView(icons).slice(&Trade::ImageData2D::size), offsets); CORRADE_INTERNAL_ASSERT(flushRange); Containers::StridedArrayView3D<Color4ub> dst = textLayerShared.glyphCache().image().pixels<Color4ub>(); for(std::size_t i = 0; i != icons.size(); ++i) { Containers::StridedArrayView3D<const Color4ub> src = icons[i].pixels<Color4ub>(); Utility::copy(src, dst.sliceSize( {std::size_t(offsets[i].z()), std::size_t(offsets[i].y()), std::size_t(offsets[i].x())}, src.size())); textLayerShared.glyphCache().addGlyph(iconFontId, i, {}, offsets[i].z(), Range2Di::fromSize(offsets[i].xy(), icons[i].size())); } textLayerShared.glyphCache().flushImage(*flushRange);
Finally create a FontHandle for the new set of glyphs using TextLayer::1.0f
if you want to draw the icons in their original pixel size. the Text crispness and DPI awareness section below for additional considerations. The font handle can then be referenced from the style or passed directly to createGlyph().
Ui::FontHandle iconFontHandle = textLayerShared.addInstancelessFont(iconFontId, 1.0f); … /* Create a glyph from containing icon at offset 3 in the `icons` array above */ textLayer.createGlyph(…, 3, iconFontHandle, …);
See Text::
Updating text data
Any created text or single glyph can be subsequently updated with setText() and setGlyph(). These functions take the same arguments and behave the same as create() and createGlyph(). Internally there's no distinction between a text and a single glyph, so a text can be safely changed to just a glyph and vice versa.
Arbitrary text and glyph transformation
By constructing the layer with TextLayerFlag::
Ui::TextLayerGL& textLayer = ui.setLayerInstance( Containers::pointer<Ui::TextLayerGL>(ui.createLayer(), textLayerShared, Ui::TextLayerFlag::Transformable));
For a more involved example, the following code will draw an analog clock with hands drawn as rotated glyphs. First, the hand is imported from an inline SVG with one of the SVG importer plugins such as the LunaSvgImporter. It'll result in a 32x256 image that's — as documented — in PixelFormat::
PluginManager::Manager<Trade::AbstractImporter> importerManager; Containers::Pointer<Trade::AbstractImporter> importer = importerManager.loadAndInstantiate("SvgImporter"); if(!importer || !importer->openData(R"( <svg version="1.1" viewBox="0 0 32 256" xmlns="http://www.w3.org/2000/svg"> <rect width="32" height="256"/> <path d="m16 0-4 16-12 224 16 16 16-16-12-224z" fill="#fff"/> </svg> )")) Fatal{} << "Uh oh"; Containers::Optional<Trade::ImageData2D> needle = importer->image2D(0);
Afterwards, similarly to the glyph cache packing code above, the image is added to the glyph cache. Just the red channel is copied because the distance field glyph cache is single-channel, and the Text::0
is added, which gets referenced later. The {-16, 16}
offset moves the glyph origin to the desired rotation center. The scale passed to TextLayer::
Vector3i offset[1]; Containers::Optional<Range3Di> flushRange = textLayer.shared().glyphCache() .atlas().add({needle->size()}, offset); CORRADE_INTERNAL_ASSERT(flushRange); Containers::StridedArrayView3D<const UnsignedByte> src = needle->pixels<Color4ub>().slice(&Color4ub::r); /* just the red channel */ Containers::StridedArrayView3D<UnsignedByte> dst = textLayer.shared().glyphCache().image().pixels<UnsignedByte>(); Utility::copy(src, dst.sliceSize( {std::size_t(offset->z()), std::size_t(offset->y()), std::size_t(offset->x())}, src.size())); textLayer.shared().glyphCache().flushImage(*flushRange); UnsignedInt needleFontId = textLayer.shared().glyphCache().addFont(1); textLayer.shared().glyphCache().addGlyph(needleFontId, 0, {-16, -16}, offset->z(), Range2Di::fromSize(offset->xy(), needle->size())); Ui::FontHandle needleFont = textLayer.shared().addInstancelessFont(needleFontId, …);
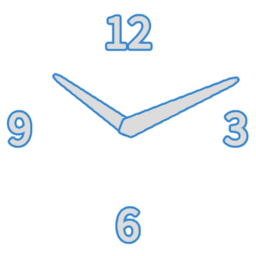
Finally, assuming style
has a regular font associated, 12-, 3-, 6- and 9-hour marks are added using various Text::needleFont
glyph 0
from above, then show a time of 10:11. The default hand rotation is at the 12-hour mark, first child is the scaled-down hand showing hours, and second, again above hours, is then minutes:
Ui::NodeHandle clock = …; textLayer.create(style, "12", Text::Alignment::TopCenter, clock); textLayer.create(style, "3", Text::Alignment::MiddleRight, clock); textLayer.create(style, "6", Text::Alignment::BottomCenter, clock); textLayer.create(style, "9", Text::Alignment::MiddleLeft, clock); Ui::NodeHandle hours = ui.createNode(clock, {}, ui.nodeSize(clock)); Ui::DataHandle hoursData = textLayer.createGlyph(style, 0, needleFont, hours); textLayer.rotate(hoursData, 360.0_degf*(10.0f + 11.0f/60.0f)/12.0f); textLayer.scale(hoursData, 0.75f); Ui::NodeHandle minutes = ui.createNode(hours, {}, ui.nodeSize(hours)); Ui::DataHandle minutesData = textLayer.createGlyph(style, 0, needleFont, minutes); textLayer.rotate(minutesData, 360.0_degf*11.0f/60.0f);
Dynamic styles
Like with BaseLayer dynamic styles, the TextLayer::
Ui::TextLayerGL::Shared textLayerShared{glyphCache, Ui::TextLayerGL::Shared::Configuration{…} .setDynamicStyleCount(10) }; Ui::TextLayerGL& textLayer = ui.setLayerInstance( Containers::pointer<Ui::TextLayerGL>(ui.createLayer(), textLayerShared)); … UnsignedInt dynamicStyleId = …; /* anything less than the dynamic style count */ textLayer.setDynamicStyle(dynamicStyleId, …); Ui::NodeHandle node = …; textLayer.create(textLayer.shared().styleCount() + dynamicStyleId, …, node);
The main use case for dynamic styles is animations, which is for the text layer implemented in the TextLayerStyleAnimator class. Again note that if you intend to directly use dynamic styles along with the animator, you should use allocateDynamicStyle() and recycleDynamicStyle() to prevent the animator from stealing dynamic styles you use elsewhere:
/* Attempt to allocate a dynamic style ID, if available */ Containers::Optional<UnsignedInt> dynamicStyleId = textLayer.allocateDynamicStyle(); if(!dynamicStyleId) { … } /* Populate it, use */ textLayer.setDynamicStyle(*dynamicStyleId, …); … /* Once not used anymore, recycle the ID again */ textLayer.recycleDynamicStyle(*dynamicStyleId);
Style transition based on input events
Like with BaseLayer, it's possible to configure TextLayer to perform automatic style transitions based on input events, such as highlighting on hover or press. See the BaseLayer documentation for style transition for a detailed example, the interfaces are the same between the two.
Editable text
By default, the text layer shapes the input text into glyphs, throwing away the input text and all its properties like language or direction, and remembering just which glyphs to render at which positions. As majority of text in user interfaces is non-editable and often only set, never queried back, there's no need to implicitly store such info.
To make editable text, first a style describing how cursor and selection looks like needs to be configured. As both the cursor and the selection are rectangular shapes, there's a single editing style definition, and then it's specified which of them are used for cursors and which for selection. By default there are no editing styles and their desired count has to be specified via TextLayer::
Ui::TextLayerGL::Shared textLayerShared{glyphCache, Ui::TextLayer::Shared::Configuration{3} .setEditingStyleCount(2) };
Style data is then supplied with TextLayer::0
for a cursor, expanding one UI unit on each side from the cursor position, and a blue selection style 1
, tightly wrapping the glyph rectangle:
textLayerShared.setEditingStyle(Ui::TextLayerCommonEditingStyleUniform{}, { Ui::TextLayerEditingStyleUniform{} /* 0 */ .setBackgroundColor(0xa5c9ea_rgbf), Ui::TextLayerEditingStyleUniform{} /* 1 */ .setBackgroundColor(0x2f83cc_rgbf), }, {}, { /* Begin, top, end, bottom */ {1.0f, 0.0f, 1.0f, 0.0f}, /* 0 */ {} /* 1 */ });
After that, the editing styles need to be referenced from the regular styles. This is done with the eighth and ninth argument to TextLayer::-1
if given style shouldn't have a cursor / selection style assigned. If either of those arguments is left empty, it's the same as if it'd have -1
for all styles. Here style 0
is a regular non-editing style, 1
is a style with both a cursor and selection style, and 2
has just a selection, for example if a certain label is meant to be just selectable but not directly editable:
textLayerShared.setStyle(…, { Ui::TextLayerStyleUniform{} /* 0 */ …, Ui::TextLayerStyleUniform{} /* 1 */ …, Ui::TextLayerStyleUniform{} /* 2 */ …, }, {…}, {…}, {}, {}, {}, { -1, /* Style 0 uses no cursor style */ 0, /* Style 1 uses editing style 0 for cursor */ -1 /* Style 2 uses no cursor style */ }, { -1, /* Style 0 uses no selection style */ 1, /* Style 1 uses editing style 1 for selection */ 1 /* Style 2 uses editing style 1 for selection */ }, {});
With the editing style set up, an editable text can be made by passing TextDataFlag::
Ui::NodeHandle node = …; Ui::DataHandle text = textLayer.create(…, "Hello world!", {}, Ui::TextDataFlag::Editable, node); textLayer.setCursor(text, 7, 4); /* Selecting "o w" with cursor at byte 7 */
Contents of an editable text are then subsequently queryable with text(). The updateText() function can perform incremental updates in addition to cursor movement and the editText() API is for higher-level operations with UTF-8 and text directionality awareness. This function is also what is called on actual key and text input events, described in Reacting to focus, pointer and keyboard input events below.
Cursor and selection color, selection text style
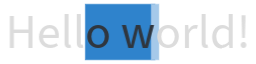
Apart from the selection / cursor rectangle color, set with TextLayerEditingStyleUniform::3
, which is then referenced from the selection editing style, and which makes the selected text darker:
textLayerShared.setStyle(…, { Ui::TextLayerStyleUniform{} /* 0 */ …, Ui::TextLayerStyleUniform{} /* 1 */ …, Ui::TextLayerStyleUniform{} /* 2 */ …, Ui::TextLayerStyleUniform{} /* 3 */ .setColor(0x2f363f_rgbf), }, …); textLayerShared.setEditingStyle(…, {…}, { -1, /* (Cursor) style 0 doesn't override any style for text selection */ 3 /* (Selection) style 1 uses style 3 for text selection */ }, {…});
Note that only the TextLayerCommonStyleUniform is used from the style override, not font, alignment or other properties.
Cursor and selection rectangle padding
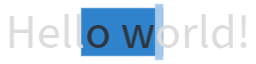
Padding used in the snippets above was only horizontal, but vertical padding can be used for example to achieve better optical alignment of both the text itself and the selection rectangle inside an edit box, or to make a cursor stand out more. Horizontal padding also doesn't necessarily have to be symmetrical. For the cursor it's often desirable to have it shifted towards the typing direction. Here it's still two units wide, but is shifted to the right for Text::
textLayerShared.setEditingStyle(…, {…}, {}, { /* Begin, top, end, bottom */ {0.0f, 0.0f, 2.0f, 0.0f}, /* (Cursor) style 0 */ {1.0f, -1.0f, 1.0f, -1.0f} /* (Selection) style 1 */ });
Rounded corners and edge smoothness
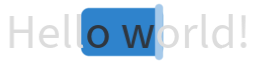
TextLayerEditingStyleUniform::
textLayerShared.setEditingStyle(…, { Ui::TextLayerEditingStyleUniform{} /* (Cursor) style 0 */ .setBackgroundColor(0xa5c9ea_rgbf) .setCornerRadius(1.0f), Ui::TextLayerEditingStyleUniform{} /* (Selection) style 1 */ .setBackgroundColor(0x2f83cc_rgbf) .setCornerRadius(2.0f), }, {…}, {…});
Reacting to focus, pointer and keyboard input events
Assuming key and text input events are propagated to the UI from the application as described in the AbstractUserInterface documentation, if a text with TextDataFlag::
Ui::NodeHandle node = ui.createNode(…, Ui::NodeFlag::Focusable); Ui::DataHandle text = textLayer.create(…, Ui::TextDataFlag::Editable, node);
For proper visual feedback on focus and blur of a particular widget containing the editable text it's recommended to implement also the toFocusedOut
and toFocusedOver
state transitions in Shared::
Implementing virtual keyboards
Besides key and text input events coming from the OS, it's possible to synthesize them to implement a virtual keyboard, for example by hooking to pointer presses using EventLayer::
An important thing to consider is that, by default, pressing outside of a node that's currently focused will blur it, which ultimately means that when using the virtual keyboard, you'd lose focus of the input field after each key press. This behavior can be disabled for a node and all its children with NodeFlag::
Ui::NodeHandle keyboard = ui.createNode(…, Ui::NodeFlag::NoBlur); Ui::NodeHandle keyQ = ui.createNode(keyboard, …); eventLayer.onPress(keyQ, [&ui]{ Ui::TextInputEvent event{{}, "q"}; ui.textInputEvent(event); }); Ui::NodeHandle keyW = ui.createNode(keyboard, …); eventLayer.onPress(keyW, [&ui]{ Ui::TextInputEvent event{{}, "w"}; ui.textInputEvent(event); }); … Ui::NodeHandle keyBackspace = ui.createNode(keyboard, …); eventLayer.onPress(keyBackspace, [&ui]{ Ui::KeyEvent event{{}, Ui::Key::Backspace, {}}; ui.keyPressEvent(event); }); …
Generally you'd synthesize a TextInputEvent for any key that inserts text, and KeyEvent for keys that perform cursor movement or deletion. Uppercase can be implemented for example by having the virtual Shift key toggle a boolean, which the EventLayer::"q"
or "Q"
. Or pressing the Shift key toggles visibility of an entirely different key set for uppercase letters, completely with different labels for those.
Dynamic editing styles
Dynamic styles, described above, have also variants that include cursor and selection styles. Depending on the subset of editing styles you want to have for a particular dynamic style, use setDynamicStyleWithCursor(), setDynamicStyleWithSelection() or setDynamicStyleWithCursorSelection() instead of setDynamicStyle().
Debug layer integration
When using DebugLayer node highlighting, this layer inherits debug integration from the AbstractVisualLayer. See its documentation for more information. If DebugLayerSource::
Node {0x12, 0x1} Nested at level 2 with 0 direct children Data {0x0, 0x1} from layer {0x8, 0x1} Text with style Label (3) Color: ▓▓ #2f83ccff Padding: 4.5
Base classes
- class AbstractVisualLayer new in Git master
- Base for visual data layers.
Derived classes
- class TextLayerGL new in Git master
- OpenGL implementation of the text layer.
Public types
- class DebugIntegration
- Debug layer integration.
- class Shared
- Shared state for the text layer.
Public functions
- auto shared() -> Shared&
- Shared state used by this layer.
- auto shared() const -> const Shared&
- auto flags() const -> TextLayerFlags
- Layer flags.
- auto assignAnimator(TextLayerStyleAnimator& animator) -> TextLayer&
- Assign a style animator to this layer.
- auto defaultStyleAnimator() const -> TextLayerStyleAnimator*
- Default style animator for this layer.
- auto setDefaultStyleAnimator(TextLayerStyleAnimator* animator) -> TextLayer&
- Set a default style animator for this layer.
-
auto dynamicStyleUniforms() const -> Containers::
ArrayView<const TextLayerStyleUniform> - Dynamic style uniforms.
-
auto dynamicStyleFonts() const -> Containers::
StridedArrayView1D<const FontHandle> - Dynamic style fonts.
-
auto dynamicStyleAlignments() const -> Containers::
StridedArrayView1D<const Text:: Alignment> - Dynamic style alignments.
-
auto dynamicStyleFeatures(UnsignedInt id) const -> Containers::
ArrayView<const TextFeatureValue> - Dynamic style font features.
-
auto dynamicStyleCursorStyles() const -> Containers::
BitArrayView - Which dynamic style have associated cursor styles.
- auto dynamicStyleCursorStyle(UnsignedInt id) const -> Int
- Dynamic style cursor style ID.
-
auto dynamicStyleSelectionStyles() const -> Containers::
BitArrayView - Which dynamic style have associated selection styles.
- auto dynamicStyleSelectionStyle(UnsignedInt id) const -> Int
- Dynamic style selection style ID.
- auto dynamicStyleSelectionStyleTextUniform(UnsignedInt) const -> Int
- Dynamic style selection style text uniform IDs.
-
auto dynamicStylePaddings() const -> Containers::
StridedArrayView1D<const Vector4> - Dynamic style paddings.
-
auto dynamicEditingStyleUniforms() const -> Containers::
ArrayView<const TextLayerEditingStyleUniform> - Dynamic editing style uniforms.
-
auto dynamicEditingStylePaddings() const -> Containers::
StridedArrayView1D<const Vector4> - Dynamic editing style paddings.
-
void setDynamicStyle(UnsignedInt id,
const TextLayerStyleUniform& uniform,
FontHandle font,
Text::
Alignment alignment, Containers:: ArrayView<const TextFeatureValue> features, const Vector4& padding) - Set a dynamic style for text only.
-
void setDynamicStyle(UnsignedInt id,
const TextLayerStyleUniform& uniform,
FontHandle font,
Text::
Alignment alignment, std:: initializer_list<TextFeatureValue> features, const Vector4& padding) -
void setDynamicStyleWithCursorSelection(UnsignedInt id,
const TextLayerStyleUniform& uniform,
FontHandle font,
Text::
Alignment alignment, Containers:: ArrayView<const TextFeatureValue> features, const Vector4& padding, const TextLayerEditingStyleUniform& cursorUniform, const Vector4& cursorPadding, const TextLayerEditingStyleUniform& selectionUniform, const Containers:: Optional<TextLayerStyleUniform>& selectionTextUniform, const Vector4& selectionPadding) - Set a dynamic style for text, cursor and selection.
-
void setDynamicStyleWithCursorSelection(UnsignedInt id,
const TextLayerStyleUniform& uniform,
FontHandle font,
Text::
Alignment alignment, std:: initializer_list<TextFeatureValue> features, const Vector4& padding, const TextLayerEditingStyleUniform& cursorUniform, const Vector4& cursorPadding, const TextLayerEditingStyleUniform& selectionUniform, const Containers:: Optional<TextLayerStyleUniform>& selectionTextUniform, const Vector4& selectionPadding) -
void setDynamicStyleWithCursor(UnsignedInt id,
const TextLayerStyleUniform& uniform,
FontHandle font,
Text::
Alignment alignment, Containers:: ArrayView<const TextFeatureValue> features, const Vector4& padding, const TextLayerEditingStyleUniform& cursorUniform, const Vector4& cursorPadding) - Set a dynamic style for text and cursor only.
-
void setDynamicStyleWithCursor(UnsignedInt id,
const TextLayerStyleUniform& uniform,
FontHandle font,
Text::
Alignment alignment, std:: initializer_list<TextFeatureValue> features, const Vector4& padding, const TextLayerEditingStyleUniform& cursorUniform, const Vector4& cursorPadding) -
void setDynamicStyleWithSelection(UnsignedInt id,
const TextLayerStyleUniform& uniform,
FontHandle font,
Text::
Alignment alignment, Containers:: ArrayView<const TextFeatureValue> features, const Vector4& padding, const TextLayerEditingStyleUniform& selectionUniform, const Containers:: Optional<TextLayerStyleUniform>& selectionTextUniform, const Vector4& selectionPadding) - Set a dynamic style for text and selection only.
-
void setDynamicStyleWithSelection(UnsignedInt id,
const TextLayerStyleUniform& uniform,
FontHandle font,
Text::
Alignment alignment, std:: initializer_list<TextFeatureValue> features, const Vector4& padding, const TextLayerEditingStyleUniform& selectionUniform, const Containers:: Optional<TextLayerStyleUniform>& selectionTextUniform, const Vector4& selectionPadding) -
auto create(UnsignedInt style,
Containers::
StringView text, const TextProperties& properties, TextDataFlags flags = {}, NodeHandle node = NodeHandle:: Null) -> DataHandle - Create a text.
-
auto create(UnsignedInt style,
Containers::
StringView text, const TextProperties& properties, NodeHandle node) -> DataHandle -
template<class StyleIndex>auto create(StyleIndex style, Containers::
StringView text, const TextProperties& properties, TextDataFlags flags = {}, NodeHandle node = NodeHandle:: Null) -> DataHandle - Create a text with a style index in a concrete enum type.
-
template<class StyleIndex>auto create(StyleIndex style, Containers::
StringView text, const TextProperties& properties, NodeHandle node) -> DataHandle -
auto createGlyph(UnsignedInt style,
UnsignedInt glyph,
const TextProperties& properties,
NodeHandle node = NodeHandle::
Null) -> DataHandle - Create a single glyph.
-
template<class StyleIndex>auto createGlyph(StyleIndex style, UnsignedInt glyph, const TextProperties& properties, NodeHandle node = NodeHandle::
Null) -> DataHandle - Create a single glyph with a style index in a concrete enum type.
-
template<class GlyphIndex>auto createGlyph(UnsignedInt style, GlyphIndex glyph, const TextProperties& properties, NodeHandle node = NodeHandle::
Null) -> DataHandle - Create a single glyph with a glyph ID in a concrete enum type.
-
template<class StyleIndex, class GlyphIndex>auto createGlyph(StyleIndex style, GlyphIndex glyph, const TextProperties& properties, NodeHandle node = NodeHandle::
Null) -> DataHandle - Create a single glyph with a style index and glyph ID in a concrete enum type.
- void remove(DataHandle handle)
- Remove a text.
- void remove(LayerDataHandle handle)
- Remove a text assuming it belongs to this layer.
- auto flags(DataHandle handle) const -> TextDataFlags
- Text flags.
- auto flags(LayerDataHandle handle) const -> TextDataFlags
- Text flags assuming it belongs to this layer.
- auto glyphCount(DataHandle handle) const -> UnsignedInt
- Text glyph count.
- auto glyphCount(LayerDataHandle handle) const -> UnsignedInt
- Text glyph count assuming it belongs to this layer.
- auto size(DataHandle handle) const -> Vector2
- Size of the laid out text.
- auto size(LayerDataHandle handle) const -> Vector2
- Size of the laid out text assuming it belongs to this layer.
-
auto cursor(DataHandle handle) const -> Containers::
Pair<UnsignedInt, UnsignedInt> - Cursor and selection position in an editable text.
-
auto cursor(LayerDataHandle handle) const -> Containers::
Pair<UnsignedInt, UnsignedInt> - Cursor and selection position in an editable text assuming it belongs to this layer.
- void setCursor(DataHandle handle, UnsignedInt position, UnsignedInt selection)
- Set cursor position and selection in an editable text.
- void setCursor(DataHandle handle, UnsignedInt position)
- Set cursor position in an editable text.
- void setCursor(LayerDataHandle handle, UnsignedInt position, UnsignedInt selection)
- Set cursor position and selection in an editable text assuming it belongs to this layer.
- void setCursor(LayerDataHandle handle, UnsignedInt position)
- Set cursor position in an editable text assuming it belongs to this layer.
- auto textProperties(DataHandle handle) const -> TextProperties
- Properties used for shaping an editable text.
- auto textProperties(LayerDataHandle handle) const -> TextProperties
- Properties used for shaping an editable text assuming it belongs to this layer.
-
auto text(DataHandle handle) const -> Containers::
StringView - Contents of an editable text.
-
auto text(LayerDataHandle handle) const -> Containers::
StringView - Contents of an editable text assuming it belongs to this layer.
-
void setText(DataHandle handle,
Containers::
StringView text, const TextProperties& properties) - Set text.
-
void setText(DataHandle handle,
Containers::
StringView text, const TextProperties& properties, TextDataFlags flags) - Set text with different flags.
-
void setText(LayerDataHandle handle,
Containers::
StringView text, const TextProperties& properties) - Set text assuming it belongs to this layer.
-
void setText(LayerDataHandle handle,
Containers::
StringView text, const TextProperties& properties, TextDataFlags flags) - Set text with different flags assuming it belongs to this layer.
-
void updateText(DataHandle handle,
UnsignedInt removeOffset,
UnsignedInt removeSize,
UnsignedInt insertOffset,
Containers::
StringView insertText, UnsignedInt cursor, UnsignedInt selection) - Update text, cursor position and selection in an editable text.
-
void updateText(DataHandle handle,
UnsignedInt removeOffset,
UnsignedInt removeSize,
UnsignedInt insertOffset,
Containers::
StringView insertText, UnsignedInt cursor) - Update text and cursor position in an editable text.
-
void updateText(LayerDataHandle handle,
UnsignedInt removeOffset,
UnsignedInt removeSize,
UnsignedInt insertOffset,
Containers::
StringView insertText, UnsignedInt cursor, UnsignedInt selection) - Update text, cursor position and selection in an editable text assuming it belongs to this layer.
-
void updateText(LayerDataHandle handle,
UnsignedInt removeOffset,
UnsignedInt removeSize,
UnsignedInt insertOffset,
Containers::
StringView insertText, UnsignedInt cursor) - Update text and cursor position in an editable text.
-
void editText(DataHandle handle,
TextEdit edit,
Containers::
StringView insert) - Edit text at current cursor position.
-
void editText(LayerDataHandle handle,
TextEdit edit,
Containers::
StringView insert) - Edit text at current cursor position assuming it belongs to this layer.
- void setGlyph(DataHandle handle, UnsignedInt glyph, const TextProperties& properties)
- Set a single glyph.
-
template<class GlyphIndex>void setGlyph(DataHandle handle, GlyphIndex glyph, const TextProperties& properties)
- Set a single glyph with a glyph ID in a concrete enum type.
- void setGlyph(LayerDataHandle handle, UnsignedInt glyph, const TextProperties& properties)
- Set a single glyph assuming it belongs to this layer.
-
template<class GlyphIndex>void setGlyph(LayerDataHandle handle, GlyphIndex glyph, const TextProperties& properties)
- Set a single glyph with a glyph ID in a concrete enum type assuming it belongs to this layer.
- auto color(DataHandle handle) const -> Color4
- Custom text base color.
- auto color(LayerDataHandle handle) const -> Color4
- Custom text base color assuming it belongs to this layer.
- void setColor(DataHandle handle, const Color4& color)
- Set custom text base color.
- void setColor(LayerDataHandle handle, const Color4& color)
- Set custom text base color assuming it belongs to this layer.
- auto padding(DataHandle handle) const -> Vector4
- Custom text padding.
- auto padding(LayerDataHandle handle) const -> Vector4
- Custom text padding assuming it belongs to this layer.
- void setPadding(DataHandle handle, const Vector4& padding)
- Set custom text padding.
- void setPadding(LayerDataHandle handle, const Vector4& padding)
- Set custom text padding assuming it belongs to this layer.
- void setPadding(DataHandle handle, Float padding)
- Set custom text padding with all edges having the same value.
- void setPadding(LayerDataHandle handle, Float padding)
- Set custom text padding with all edges having the same value assuming it belongs to this layer.
-
auto transformation(DataHandle handle) const -> Containers::
Pair<Vector2, Complex> - Custom text transformation.
-
auto transformation(LayerDataHandle handle) const -> Containers::
Pair<Vector2, Complex> - Custom text transformation.
- void setTransformation(DataHandle handle, const Vector2& translation, const Complex& rotation, Float scaling)
- Set custom text transformation.
- void setTransformation(LayerDataHandle handle, const Vector2& translation, const Complex& rotation, Float scaling)
- Set custom text transformation.
- void setTransformation(DataHandle handle, const Vector2& translation, Rad rotation, Float scaling)
- Set custom text transformation.
- void setTransformation(LayerDataHandle handle, const Vector2& translation, Rad rotation, Float scaling)
- Set custom text transformation.
- void translate(DataHandle handle, const Vector2& translation)
- Add to custom text translation.
- void translate(LayerDataHandle handle, const Vector2& translation)
- Add to custom text translation.
- void rotate(DataHandle handle, const Complex& rotation)
- Add to custom text rotation.
- void rotate(LayerDataHandle handle, const Complex& rotation)
- Add to custom text rotation.
- void rotate(DataHandle handle, Rad rotation)
- Add to custom text rotation.
- void rotate(LayerDataHandle handle, Rad rotation)
- Add to custom text rotation.
- void scale(DataHandle handle, Float scaling)
- Update custom text scaling.
- void scale(LayerDataHandle handle, Float scaling)
- Update custom text scaling.
Function documentation
Shared& Magnum:: Ui:: TextLayer:: shared()
Shared state used by this layer.
Reference to the instance passed to TextLayerGL::
TextLayerFlags Magnum:: Ui:: TextLayer:: flags() const
Layer flags.
TextLayer& Magnum:: Ui:: TextLayer:: assignAnimator(TextLayerStyleAnimator& animator)
Assign a style animator to this layer.
Returns | Reference to self (for method chaining) |
---|
Expects that Shared::animator
wasn't passed to assignAnimator() on any layer yet. On the other hand, it's possible to associate multiple different animators with the same layer.
TextLayerStyleAnimator* Magnum:: Ui:: TextLayer:: defaultStyleAnimator() const
Default style animator for this layer.
If a style animator hasn't been set, returns nullptr
. If not nullptr
, the returned animator is guaranteed to be assigned to this layer, i.e. that BaseLayerStyleAnimator::
TextLayer& Magnum:: Ui:: TextLayer:: setDefaultStyleAnimator(TextLayerStyleAnimator* animator)
Set a default style animator for this layer.
Returns | Reference to self (for method chaining) |
---|
Makes animator
used in style transitions in response to events. Expects that animator
is either nullptr
or is already assigned to this layer, i.e. that assignAnimator() was called on this layer with animator
before. Calling this function again with a different animator or with nullptr
replaces the previous one.
Containers:: ArrayView<const TextLayerStyleUniform> Magnum:: Ui:: TextLayer:: dynamicStyleUniforms() const
Dynamic style uniforms.
Size of the returned view is at least Shared::true
, size of the returned view is three times Shared::
Containers:: StridedArrayView1D<const FontHandle> Magnum:: Ui:: TextLayer:: dynamicStyleFonts() const
Dynamic style fonts.
Size of the returned view is Shared::
Containers:: StridedArrayView1D<const Text:: Alignment> Magnum:: Ui:: TextLayer:: dynamicStyleAlignments() const
Dynamic style alignments.
Size of the returned view is Shared::
Containers:: ArrayView<const TextFeatureValue> Magnum:: Ui:: TextLayer:: dynamicStyleFeatures(UnsignedInt id) const
Dynamic style font features.
Expects that the id
is less than Shared::
Containers:: BitArrayView Magnum:: Ui:: TextLayer:: dynamicStyleCursorStyles() const
Which dynamic style have associated cursor styles.
Size of the returned view is Shared::
Int Magnum:: Ui:: TextLayer:: dynamicStyleCursorStyle(UnsignedInt id) const
Dynamic style cursor style ID.
Expects that the id
is less than Shared::-1
, otherwise the returned index is a constant calculated from id
and points to the dynamicEditingStyleUniforms() and dynamicEditingStylePaddings() arrays.
Containers:: BitArrayView Magnum:: Ui:: TextLayer:: dynamicStyleSelectionStyles() const
Which dynamic style have associated selection styles.
Size of the returned view is Shared::
Int Magnum:: Ui:: TextLayer:: dynamicStyleSelectionStyle(UnsignedInt id) const
Dynamic style selection style ID.
Expects that the id
is less than Shared::-1
, otherwise the returned index is a constant calculated from id
and points to the dynamicEditingStyleUniforms() and dynamicEditingStylePaddings() arrays. Use dynamicStyleSelectionStyleTextUniform(UnsignedInt) const to retrieve ID of the uniform override applied to selected text.
Int Magnum:: Ui:: TextLayer:: dynamicStyleSelectionStyleTextUniform(UnsignedInt) const
Dynamic style selection style text uniform IDs.
Expects that the id
is less than Shared::-1
, otherwise the returned index is a constant calculated based on id
and points to the dynamicStyleUniforms() array.
In particular, contrary to the styles specified with Shared::
Containers:: StridedArrayView1D<const Vector4> Magnum:: Ui:: TextLayer:: dynamicStylePaddings() const
Dynamic style paddings.
Size of the returned view is Shared::
Containers:: ArrayView<const TextLayerEditingStyleUniform> Magnum:: Ui:: TextLayer:: dynamicEditingStyleUniforms() const
Dynamic editing style uniforms.
Size of the returned view is twice Shared::
Containers:: StridedArrayView1D<const Vector4> Magnum:: Ui:: TextLayer:: dynamicEditingStylePaddings() const
Dynamic editing style paddings.
Size of the returned view is twice Shared::
void Magnum:: Ui:: TextLayer:: setDynamicStyle(UnsignedInt id,
const TextLayerStyleUniform& uniform,
FontHandle font,
Text:: Alignment alignment,
Containers:: ArrayView<const TextFeatureValue> features,
const Vector4& padding)
Set a dynamic style for text only.
Parameters | |
---|---|
id | Dynamic style ID |
uniform | Style uniform |
font | Font handle |
alignment | Alignment |
features | Font features to use |
padding | Padding inside the node in order left, top, right, bottom |
Expects that the id
is less than Shared::font
is either FontHandle::alignment
is not *GlyphBounds
as the implementation can only align based on font metrics and cursor position, not actual glyph bounds. Shared::id
is then a style index that can be passed to create(), createGlyph() or setStyle() in order to use this style. Compared to Shared::
Calling this function causes LayerState::padding
changed, LayerState::font
, alignment
or features
doesn't cause LayerState::font
, alignment
and features
get only used in the following call to create(), createGlyph(), setText() or setGlyph().
This function doesn't set any cursor or selection style, meaning they won't be shown at all if using this style for a TextDataFlag::
void Magnum:: Ui:: TextLayer:: setDynamicStyle(UnsignedInt id,
const TextLayerStyleUniform& uniform,
FontHandle font,
Text:: Alignment alignment,
std:: initializer_list<TextFeatureValue> features,
const Vector4& padding)
This is an overloaded member function, provided for convenience. It differs from the above function only in what argument(s) it accepts.
void Magnum:: Ui:: TextLayer:: setDynamicStyleWithCursorSelection(UnsignedInt id,
const TextLayerStyleUniform& uniform,
FontHandle font,
Text:: Alignment alignment,
Containers:: ArrayView<const TextFeatureValue> features,
const Vector4& padding,
const TextLayerEditingStyleUniform& cursorUniform,
const Vector4& cursorPadding,
const TextLayerEditingStyleUniform& selectionUniform,
const Containers:: Optional<TextLayerStyleUniform>& selectionTextUniform,
const Vector4& selectionPadding)
Set a dynamic style for text, cursor and selection.
Parameters | |
---|---|
id | Dynamic style ID |
uniform | Style uniform |
font | Font handle |
alignment | Alignment |
features | Font features to use |
padding | Padding inside the node in order left, top, right, bottom |
cursorUniform | Style uniform for the editing cursor |
cursorPadding | Padding around the editing cursor in order left, top, right, bottom |
selectionUniform | Style uniform for the editing selection |
selectionTextUniform | Style uniform applied to selected portions of the text or Containers::uniform should be used for the selection as well |
selectionPadding | Padding around the editing selection in order left, top, right, bottom |
Expects that the id
is less than Shared::true
, font
is either FontHandle::alignment
is not *GlyphBounds
as the implementation can only align based on font metrics and cursor position, not actual glyph bounds. Shared::id
is then a style index that can be passed to create(), createGlyph() or setStyle() in order to use this style. Compared to Shared::
Calling this function causes LayerState::padding
, cursorPadding
or selectionPadding
changed, LayerState::font
, alignment
or features
doesn't cause any state flag to be set — the font
, alignment
and features
get only used in the following call to create(), createGlyph(), setText() or setGlyph().
Note that while selectionPadding
is merely a way to visually fine-tune the appearance, cursorPadding
has to be non-zero horizontally to actually be visible at all.
Use setDynamicStyleWithCursor() if showing selection is not desirable for given style, setDynamicStyleWithSelection() if showing cursor is not desirable and setDynamicStyle() for non-editable styles or editable styles where showing neither cursor nor selection is desirable, for example in widgets that aren't focused.
void Magnum:: Ui:: TextLayer:: setDynamicStyleWithCursorSelection(UnsignedInt id,
const TextLayerStyleUniform& uniform,
FontHandle font,
Text:: Alignment alignment,
std:: initializer_list<TextFeatureValue> features,
const Vector4& padding,
const TextLayerEditingStyleUniform& cursorUniform,
const Vector4& cursorPadding,
const TextLayerEditingStyleUniform& selectionUniform,
const Containers:: Optional<TextLayerStyleUniform>& selectionTextUniform,
const Vector4& selectionPadding)
This is an overloaded member function, provided for convenience. It differs from the above function only in what argument(s) it accepts.
void Magnum:: Ui:: TextLayer:: setDynamicStyleWithCursor(UnsignedInt id,
const TextLayerStyleUniform& uniform,
FontHandle font,
Text:: Alignment alignment,
Containers:: ArrayView<const TextFeatureValue> features,
const Vector4& padding,
const TextLayerEditingStyleUniform& cursorUniform,
const Vector4& cursorPadding)
Set a dynamic style for text and cursor only.
Parameters | |
---|---|
id | Dynamic style ID |
uniform | Style uniform |
font | Font handle |
alignment | Alignment |
features | Font features to use |
padding | Padding inside the node in order left, top, right, bottom |
cursorUniform | Style uniform for the editing cursor |
cursorPadding | Padding around the editing cursor in order left, top, right, bottom |
Compared to setDynamicStyleWithCursorSelection(UnsignedInt, const TextLayerStyleUniform&, FontHandle, Text::
void Magnum:: Ui:: TextLayer:: setDynamicStyleWithCursor(UnsignedInt id,
const TextLayerStyleUniform& uniform,
FontHandle font,
Text:: Alignment alignment,
std:: initializer_list<TextFeatureValue> features,
const Vector4& padding,
const TextLayerEditingStyleUniform& cursorUniform,
const Vector4& cursorPadding)
This is an overloaded member function, provided for convenience. It differs from the above function only in what argument(s) it accepts.
void Magnum:: Ui:: TextLayer:: setDynamicStyleWithSelection(UnsignedInt id,
const TextLayerStyleUniform& uniform,
FontHandle font,
Text:: Alignment alignment,
Containers:: ArrayView<const TextFeatureValue> features,
const Vector4& padding,
const TextLayerEditingStyleUniform& selectionUniform,
const Containers:: Optional<TextLayerStyleUniform>& selectionTextUniform,
const Vector4& selectionPadding)
Set a dynamic style for text and selection only.
Parameters | |
---|---|
id | Dynamic style ID |
uniform | Style uniform |
font | Font handle |
alignment | Alignment |
features | Font features to use |
padding | Padding inside the node in order left, top, right, bottom |
selectionUniform | Style uniform for the editing selection |
selectionTextUniform | Style uniform applied to selected portions of the text or Containers::uniform should be used for the selection as well |
selectionPadding | Padding around the editing selection in order left, top, right, bottom |
Compared to setDynamicStyleWithCursorSelection(UnsignedInt, const TextLayerStyleUniform&, FontHandle, Text::
void Magnum:: Ui:: TextLayer:: setDynamicStyleWithSelection(UnsignedInt id,
const TextLayerStyleUniform& uniform,
FontHandle font,
Text:: Alignment alignment,
std:: initializer_list<TextFeatureValue> features,
const Vector4& padding,
const TextLayerEditingStyleUniform& selectionUniform,
const Containers:: Optional<TextLayerStyleUniform>& selectionTextUniform,
const Vector4& selectionPadding)
This is an overloaded member function, provided for convenience. It differs from the above function only in what argument(s) it accepts.
DataHandle Magnum:: Ui:: TextLayer:: create(UnsignedInt style,
Containers:: StringView text,
const TextProperties& properties,
TextDataFlags flags = {},
NodeHandle node = NodeHandle:: Null)
Create a text.
Parameters | |
---|---|
style | Style index |
text | Text to render |
properties | Text properties |
flags | Flags |
node | Node to attach to |
Returns | New data handle |
Expects that Shared::style
is less than Shared::flags
don't contain TextDataFlag::style
. If TextProperties::style
or from properties
, is expected to have a font instance. Instance-less fonts can be only used to create single glyphs (such as various icons or images) with createGlyph().
If flags
contain TextDataFlag::text
and properties
are remembered and subsequently accessible through text() and textProperties(), cursor() position and selection are both set to text
size. Currently, for editable text, the properties
are expected to have empty TextProperties::
DataHandle Magnum:: Ui:: TextLayer:: create(UnsignedInt style,
Containers:: StringView text,
const TextProperties& properties,
NodeHandle node)
This is an overloaded member function, provided for convenience. It differs from the above function only in what argument(s) it accepts.
template<class StyleIndex>
DataHandle Magnum:: Ui:: TextLayer:: create(StyleIndex style,
Containers:: StringView text,
const TextProperties& properties,
TextDataFlags flags = {},
NodeHandle node = NodeHandle:: Null)
Create a text with a style index in a concrete enum type.
Casts style
to UnsignedInt and delegates to create(UnsignedInt, Containers::
template<class StyleIndex>
DataHandle Magnum:: Ui:: TextLayer:: create(StyleIndex style,
Containers:: StringView text,
const TextProperties& properties,
NodeHandle node)
This is an overloaded member function, provided for convenience. It differs from the above function only in what argument(s) it accepts.
DataHandle Magnum:: Ui:: TextLayer:: createGlyph(UnsignedInt style,
UnsignedInt glyph,
const TextProperties& properties,
NodeHandle node = NodeHandle:: Null)
Create a single glyph.
Parameters | |
---|---|
style | Style index |
glyph | Glyph ID to render |
properties | Text properties |
node | Node to attach to |
Returns | New data handle |
Expects that Shared::style
is less than Shared::style
. If TextProperties::glyph
is expected to be less than Text::
Compared to create(), the glyph is aligned according to TextProperties::*Start
or *End
, it's converted to an appropriate *Left
or *Right
value based on TextProperties::
template<class StyleIndex>
DataHandle Magnum:: Ui:: TextLayer:: createGlyph(StyleIndex style,
UnsignedInt glyph,
const TextProperties& properties,
NodeHandle node = NodeHandle:: Null)
Create a single glyph with a style index in a concrete enum type.
Casts style
to UnsignedInt and delegates to createGlyph(UnsignedInt, UnsignedInt, const TextProperties&, NodeHandle).
template<class GlyphIndex>
DataHandle Magnum:: Ui:: TextLayer:: createGlyph(UnsignedInt style,
GlyphIndex glyph,
const TextProperties& properties,
NodeHandle node = NodeHandle:: Null)
Create a single glyph with a glyph ID in a concrete enum type.
Casts glyph
to UnsignedInt and delegates to createGlyph(UnsignedInt, UnsignedInt, const TextProperties&, NodeHandle).
template<class StyleIndex, class GlyphIndex>
DataHandle Magnum:: Ui:: TextLayer:: createGlyph(StyleIndex style,
GlyphIndex glyph,
const TextProperties& properties,
NodeHandle node = NodeHandle:: Null)
Create a single glyph with a style index and glyph ID in a concrete enum type.
Casts style
and glyph
to UnsignedInt and delegates to createGlyph(UnsignedInt, UnsignedInt, const TextProperties&, NodeHandle).
void Magnum:: Ui:: TextLayer:: remove(DataHandle handle)
Remove a text.
Delegates to AbstractLayer::
void Magnum:: Ui:: TextLayer:: remove(LayerDataHandle handle)
Remove a text assuming it belongs to this layer.
Delegates to AbstractLayer::
TextDataFlags Magnum:: Ui:: TextLayer:: flags(DataHandle handle) const
Text flags.
Expects that handle
is valid. Note that, to avoid implementation complexity, the flags can be only specified in create() or setText(DataHandle, Containers::
TextDataFlags Magnum:: Ui:: TextLayer:: flags(LayerDataHandle handle) const
Text flags assuming it belongs to this layer.
Like flags(DataHandle) const but without checking that handle
indeed belongs to this layer. See its documentation for more information.
UnsignedInt Magnum:: Ui:: TextLayer:: glyphCount(DataHandle handle) const
Text glyph count.
Expects that handle
is valid.
UnsignedInt Magnum:: Ui:: TextLayer:: glyphCount(LayerDataHandle handle) const
Text glyph count assuming it belongs to this layer.
Expects that handle
is valid.
Vector2 Magnum:: Ui:: TextLayer:: size(DataHandle handle) const
Size of the laid out text.
Expects that handle
is valid. For text laid out with create() or setText() the size is derived from ascent, descent and advances of individual glyphs, not from actual area of the glyphs being drawn, as that may not be known at that time. For createGlyph() or setGlyph() the size is based on the actual glyph size coming out of the glyph cache.
Text transformation, if TextLayerFlag::
Vector2 Magnum:: Ui:: TextLayer:: size(LayerDataHandle handle) const
Size of the laid out text assuming it belongs to this layer.
Like size(DataHandle) const but without checking that handle
indeed belongs to this layer. See its documentation for more information.
Containers:: Pair<UnsignedInt, UnsignedInt> Magnum:: Ui:: TextLayer:: cursor(DataHandle handle) const
Cursor and selection position in an editable text.
Expects that handle
is valid and the text was created or set with TextDataFlag::
Containers:: Pair<UnsignedInt, UnsignedInt> Magnum:: Ui:: TextLayer:: cursor(LayerDataHandle handle) const
Cursor and selection position in an editable text assuming it belongs to this layer.
Like cursor(DataHandle) const but without checking that handle
indeed belongs to this layer. See its documentation for more information.
void Magnum:: Ui:: TextLayer:: setCursor(DataHandle handle,
UnsignedInt position,
UnsignedInt selection)
Set cursor position and selection in an editable text.
Low-level interface for cursor positioning. See updateText() for a low-level interface to perform text modifications together with cursor positioning, use editText() to perform higher-level operations with UTF-8 and text directionality awareness.
Expects that handle
is valid and the text was created or set with TextDataFlag::position
and selection
is expected to be less or equal to text() size. The distance between the two is what's selected — if selection
is less than position
, the selection is before the cursor, if position
is less than selection
, the selection is after the cursor. If they're the same, there's no selection. No UTF-8 sequence boundary adjustment is done for either of the two, i.e. it's possible to move the cursor or selection inside a multi-byte UTF-8 sequence.
Calling this function causes LayerState::removeSize
and insertText
size are both 0
and cursor
is equal to cursor().
void Magnum:: Ui:: TextLayer:: setCursor(DataHandle handle,
UnsignedInt position)
Set cursor position in an editable text.
Same as calling setCursor(DataHandle, UnsignedInt, UnsignedInt) with position
passed to both position
and selection
, i.e. with nothing selected.
void Magnum:: Ui:: TextLayer:: setCursor(LayerDataHandle handle,
UnsignedInt position,
UnsignedInt selection)
Set cursor position and selection in an editable text assuming it belongs to this layer.
Like setCursor(DataHandle, UnsignedInt, UnsignedInt) but without checking that handle
indeed belongs to this layer. See its documentation for more information.
void Magnum:: Ui:: TextLayer:: setCursor(LayerDataHandle handle,
UnsignedInt position)
Set cursor position in an editable text assuming it belongs to this layer.
Same as calling setCursor(LayerDataHandle, UnsignedInt, UnsignedInt) with position
passed to both position
and selection
, i.e. with nothing selected.
TextProperties Magnum:: Ui:: TextLayer:: textProperties(DataHandle handle) const
Properties used for shaping an editable text.
Expects that handle
is valid and the text was created with TextDataFlag::handle
, except for TextProperties::
TextProperties Magnum:: Ui:: TextLayer:: textProperties(LayerDataHandle handle) const
Properties used for shaping an editable text assuming it belongs to this layer.
Like textProperties(DataHandle) const but without checking that handle
indeed belongs to this layer. See its documentation for more information.
Containers:: StringView Magnum:: Ui:: TextLayer:: text(DataHandle handle) const
Contents of an editable text.
Expects that handle
is valid and the text was created or set with TextDataFlag::
Containers:: StringView Magnum:: Ui:: TextLayer:: text(LayerDataHandle handle) const
Contents of an editable text assuming it belongs to this layer.
Like text(DataHandle) const but without checking that handle
indeed belongs to this layer. See its documentation for more information.
void Magnum:: Ui:: TextLayer:: setText(DataHandle handle,
Containers:: StringView text,
const TextProperties& properties)
Set text.
Expects that handle
is valid and TextProperties::properties
, is expected to have a font instance. Instance-less fonts can be only used to set single glyphs (such as various icons or images) with setGlyph().
This function preserves existing flags(DataHandle) const for given handle
, use setText(DataHandle, Containers::
If flags(DataHandle) const contain TextDataFlag::text
and properties
are remembered and subsequently accessible through text() and textProperties(), cursor() position and selection are both set to text
size. Currently, for editable text, the properties
are expected to have empty TextProperties::
Calling this function causes LayerState::
void Magnum:: Ui:: TextLayer:: setText(DataHandle handle,
Containers:: StringView text,
const TextProperties& properties,
TextDataFlags flags)
Set text with different flags.
Like setText(DataHandle, Containers::flags
don't contain TextDataFlag::
void Magnum:: Ui:: TextLayer:: setText(LayerDataHandle handle,
Containers:: StringView text,
const TextProperties& properties)
Set text assuming it belongs to this layer.
Like setText(DataHandle, Containers::handle
indeed belongs to this layer. See its documentation for more information.
void Magnum:: Ui:: TextLayer:: setText(LayerDataHandle handle,
Containers:: StringView text,
const TextProperties& properties,
TextDataFlags flags)
Set text with different flags assuming it belongs to this layer.
Like setText(DataHandle, Containers::handle
indeed belongs to this layer. See its documentation for more information.
void Magnum:: Ui:: TextLayer:: updateText(DataHandle handle,
UnsignedInt removeOffset,
UnsignedInt removeSize,
UnsignedInt insertOffset,
Containers:: StringView insertText,
UnsignedInt cursor,
UnsignedInt selection)
Update text, cursor position and selection in an editable text.
Parameters | |
---|---|
handle | Handle which to update |
removeOffset | Offset at which to remove |
removeSize | Count of bytes to remove |
insertOffset | Offset at which to insert after the removal |
insertText | Text to insert after the removal |
cursor | Cursor position to set after the removal and subsequent insert |
selection | Selection to set after the removal and subsequent insert |
Low-level interface for text removal and insertion together with cursor positioning. See setCursor() for just cursor positioning alone, use editText() to perform higher-level operations with UTF-8 and text directionality awareness.
Expects that handle
is valid and the text was created or set with TextDataFlag::removeOffset
together with removeSize
is expected to be less or equal to text() size; insertOffset
then equal to the text size without removeSize
; cursor
and selection
then to text size without removeSize
but with insertText
size. The distance between cursor
and selection
is what's selected — if selection
is less than cursor
, the selection is before the cursor, if cursor
is less than selection
, the selection is after the cursor. If they're the same, there's no selection. No UTF-8 sequence boundary adjustment is done for any of these, i.e. it's possible to remove or insert partial multi-byte UTF-8 sequences and position the cursor inside them as well.
Calling this function causes LayerState::removeSize
and insertText
size are both 0
and cursor
is equal to cursor().
void Magnum:: Ui:: TextLayer:: updateText(DataHandle handle,
UnsignedInt removeOffset,
UnsignedInt removeSize,
UnsignedInt insertOffset,
Containers:: StringView insertText,
UnsignedInt cursor)
Update text and cursor position in an editable text.
Same as calling updateText(DataHandle, UnsignedInt, UnsignedInt, UnsignedInt, Containers::position
passed to both position
and selection
, i.e. with nothing selected.
void Magnum:: Ui:: TextLayer:: updateText(LayerDataHandle handle,
UnsignedInt removeOffset,
UnsignedInt removeSize,
UnsignedInt insertOffset,
Containers:: StringView insertText,
UnsignedInt cursor,
UnsignedInt selection)
Update text, cursor position and selection in an editable text assuming it belongs to this layer.
Like updateText(DataHandle, UnsignedInt, UnsignedInt, UnsignedInt, Containers::handle
indeed belongs to this layer. See its documentation for more information.
void Magnum:: Ui:: TextLayer:: updateText(LayerDataHandle handle,
UnsignedInt removeOffset,
UnsignedInt removeSize,
UnsignedInt insertOffset,
Containers:: StringView insertText,
UnsignedInt cursor)
Update text and cursor position in an editable text.
Same as calling updateText(DataHandle, UnsignedInt, UnsignedInt, UnsignedInt, Containers::position
passed to both position
and selection
, i.e. with nothing selected.
void Magnum:: Ui:: TextLayer:: editText(DataHandle handle,
TextEdit edit,
Containers:: StringView insert)
Edit text at current cursor position.
High-level interface for text editing, mapping to usual user interface operations. Delegates to setCursor() or updateText() internally.
Expects that handle
is valid, the text was created or set with TextDataFlag::insert
is non-empty only if appropriate edit
operation is used. See documentation of the TextEdit enum for detailed behavior of each operation.
Calling this function causes LayerState::
void Magnum:: Ui:: TextLayer:: editText(LayerDataHandle handle,
TextEdit edit,
Containers:: StringView insert)
Edit text at current cursor position assuming it belongs to this layer.
Like editText(DataHandle, TextEdit, Containers::handle
indeed belongs to this layer. See its documentation for more information.
void Magnum:: Ui:: TextLayer:: setGlyph(DataHandle handle,
UnsignedInt glyph,
const TextProperties& properties)
Set a single glyph.
Expects that handle
is valid and TextProperties::glyph
is expected to be less than Text::
Compared to setText(), the glyph is aligned according to TextProperties::
Calling this function causes LayerState::
template<class GlyphIndex>
void Magnum:: Ui:: TextLayer:: setGlyph(DataHandle handle,
GlyphIndex glyph,
const TextProperties& properties)
Set a single glyph with a glyph ID in a concrete enum type.
Casts glyph
to UnsignedInt and delegates to setGlyph(DataHandle, UnsignedInt, const TextProperties&).
void Magnum:: Ui:: TextLayer:: setGlyph(LayerDataHandle handle,
UnsignedInt glyph,
const TextProperties& properties)
Set a single glyph assuming it belongs to this layer.
Like setGlyph(DataHandle, UnsignedInt, const TextProperties&) but without checking that handle
indeed belongs to this layer. See its documentation for more information.
template<class GlyphIndex>
void Magnum:: Ui:: TextLayer:: setGlyph(LayerDataHandle handle,
GlyphIndex glyph,
const TextProperties& properties)
Set a single glyph with a glyph ID in a concrete enum type assuming it belongs to this layer.
Casts glyph
to UnsignedInt and delegates to setGlyph(LayerDataHandle, UnsignedInt, const TextProperties&).
Color4 Magnum:: Ui:: TextLayer:: color(DataHandle handle) const
Custom text base color.
Expects that handle
is valid.
Color4 Magnum:: Ui:: TextLayer:: color(LayerDataHandle handle) const
Custom text base color assuming it belongs to this layer.
Expects that handle
is valid.
void Magnum:: Ui:: TextLayer:: setColor(DataHandle handle,
const Color4& color)
Set custom text base color.
Expects that handle
is valid. The color
is expected to have premultiplied alpha. It is multiplied with TextLayerStyleUniform::0xffffffff_srgbaf
, i.e. not affecting the color coming from the style in any way.
Calling this function causes LayerState::
void Magnum:: Ui:: TextLayer:: setColor(LayerDataHandle handle,
const Color4& color)
Set custom text base color assuming it belongs to this layer.
Like setColor(DataHandle, const Color4&) but without checking that handle
indeed belongs to this layer. See its documentation for more information.
Vector4 Magnum:: Ui:: TextLayer:: padding(DataHandle handle) const
Custom text padding.
In UI units, in order left, top, right, bottom. Expects that that the layer was not created with TextLayerFlag::handle
is valid.
Vector4 Magnum:: Ui:: TextLayer:: padding(LayerDataHandle handle) const
Custom text padding assuming it belongs to this layer.
Like padding(DataHandle) const but without checking that handle
indeed belongs to this layer. See its documentation for more information.
void Magnum:: Ui:: TextLayer:: setPadding(DataHandle handle,
const Vector4& padding)
Set custom text padding.
Expects that that the layer was not created with TextLayerFlag::handle
is valid. The padding
is in UI units, in order left, top, right, bottom, and is added to the per-style padding values specified in Shared::
Calling this function causes LayerState::
void Magnum:: Ui:: TextLayer:: setPadding(LayerDataHandle handle,
const Vector4& padding)
Set custom text padding assuming it belongs to this layer.
Like setPadding(DataHandle, const Vector4&) but without checking that handle
indeed belongs to this layer. See its documentation for more information.
void Magnum:: Ui:: TextLayer:: setPadding(DataHandle handle,
Float padding)
Set custom text padding with all edges having the same value.
Equivalent to calling setPadding(DataHandle, const Vector4&) with all four components set to padding
. See its documentation for more information.
void Magnum:: Ui:: TextLayer:: setPadding(LayerDataHandle handle,
Float padding)
Set custom text padding with all edges having the same value assuming it belongs to this layer.
Like setPadding(DataHandle, Float) but without checking that handle
indeed belongs to this layer. See its documentation for more information.
Containers:: Pair<Vector2, Complex> Magnum:: Ui:: TextLayer:: transformation(DataHandle handle) const
Custom text transformation.
Translation and clockwise rotation multiplied by uniform scaling. Use Complex::handle
is valid.
Containers:: Pair<Vector2, Complex> Magnum:: Ui:: TextLayer:: transformation(LayerDataHandle handle) const
Custom text transformation.
Like transformation(DataHandle) const but without checking that handle
indeed belongs to this layer. See its documentation for more information.
void Magnum:: Ui:: TextLayer:: setTransformation(DataHandle handle,
const Vector2& translation,
const Complex& rotation,
Float scaling)
Set custom text transformation.
Expects that the layer was created with TextLayerFlag::handle
is valid. The translation
is applied on top of how a particular Text::rotation
is interpreted with positive angle clockwise for consistency with the UI coordinate system being Y down. If rotation
is not normalized, its length multiplies scaling
. Scaling is applied first, rotation second and translation last. By default, the custom transformation is a zero translation vector, identity rotation and scaling of 1.0f
.
Rotation passed as a Complex is preferrable for cases of supplying a concrete rotation several times to minimize trigonometry calculations. Use the setTransformation(DataHandle, const Vector2&, Rad, Float) convenience overload to supply a concrete angle. Use translate(), rotate() and scale() to incrementally change individual properties.
Calling this function causes LayerState::
void Magnum:: Ui:: TextLayer:: setTransformation(LayerDataHandle handle,
const Vector2& translation,
const Complex& rotation,
Float scaling)
Set custom text transformation.
Like setTransformation(DataHandle, const Vector2&, const Complex&, Float) but without checking that handle
indeed belongs to this layer. See its documentation for more information.
void Magnum:: Ui:: TextLayer:: setTransformation(DataHandle handle,
const Vector2& translation,
Rad rotation,
Float scaling)
Set custom text transformation.
Equivalent to calling setTransformation(DataHandle, const Vector2&, const Complex&, Float) with rotation
passed as Complex::
If supplying a concrete rotation many times, passing it as a Complex to setTransformation(DataHandle, const Vector2&, const Complex&, Float) instead of using this function is preferrable to minimize trigonometry calculations.
void Magnum:: Ui:: TextLayer:: setTransformation(LayerDataHandle handle,
const Vector2& translation,
Rad rotation,
Float scaling)
Set custom text transformation.
Like setTransformation(DataHandle, const Vector2&, Rad, Float) but without checking that handle
indeed belongs to this layer. See its documentation for more information.
void Magnum:: Ui:: TextLayer:: translate(DataHandle handle,
const Vector2& translation)
Add to custom text translation.
Expects that the layer was created with TextLayerFlag::handle
is valid. The translation
is added to existing custom translation, on top of how a particular Text::
Calling this function causes LayerState::
void Magnum:: Ui:: TextLayer:: translate(LayerDataHandle handle,
const Vector2& translation)
Add to custom text translation.
Like translate(DataHandle, const Vector2&) but without checking that handle
indeed belongs to this layer. See its documentation for more information.
void Magnum:: Ui:: TextLayer:: rotate(DataHandle handle,
const Complex& rotation)
Add to custom text rotation.
Expects that the layer was created with TextLayerFlag::handle
is valid. The rotation
is interpreted with positive angle clockwise for consistency with the UI coordinate system being Y down, and is added to existing custom rotation. Use setTransformation() to reset the rotation to a concrete value. If rotation
is not normalized, its length multiplies existing custom scaling. The translation, which is applied after rotation and scaling, stays unchanged.
Rotation passed as a Complex is preferrable for cases of supplying a concrete rotation several times to minimize trigonometry calculations. Use the rotate(DataHandle, Rad) convenience overload to supply a concrete angle.
Calling this function causes LayerState::
void Magnum:: Ui:: TextLayer:: rotate(LayerDataHandle handle,
const Complex& rotation)
Add to custom text rotation.
Like rotate(DataHandle, const Complex&) but without checking that handle
indeed belongs to this layer. See its documentation for more information.
void Magnum:: Ui:: TextLayer:: rotate(DataHandle handle,
Rad rotation)
Add to custom text rotation.
Equivalent to calling rotate(DataHandle, const Complex&) with rotation
passed as Complex::
If supplying a concrete rotation many times, passing it as a Complex to rotate(DataHandle, const Complex&) instead of using this function is preferrable to minimize trigonometry calculations.
void Magnum:: Ui:: TextLayer:: rotate(LayerDataHandle handle,
Rad rotation)
Add to custom text rotation.
Like rotate(DataHandle, Rad) but without checking that handle
indeed belongs to this layer. See its documentation for more information.
void Magnum:: Ui:: TextLayer:: scale(DataHandle handle,
Float scaling)
Update custom text scaling.
Expects that the layer was created with TextLayerFlag::handle
is valid. The scaling
multiplies existing custom scaling. Use setTransformation() to reset the scaling to a concrete value. The rotation and translation, which are both applied after scaling, stay unchanged. By default, the custom scaling is 1.0f
.
Calling this function causes LayerState::
void Magnum:: Ui:: TextLayer:: scale(LayerDataHandle handle,
Float scaling)
Update custom text scaling.
Like scale(DataHandle, Float) but without checking that handle
indeed belongs to this layer. See its documentation for more information.